Computer Applications
You have a saving account in a bank with some balance amount in your account. Now, you want to perform the following tasks, as per your choice. The tasks are as under.
1. Money Deposited
2. Money Withdrawn
3. Check balance
0. To quit
Write a menu driven program to take input from the user and perform the above tasks. The program checks the balance before withdrawal and finally displays the current balance after transaction. For an incorrect choice, an appropriate message should be displayed.
Java
Java Conditional Stmts
30 Likes
Answer
import java.util.*;
public class KboatBankAccount
{
public static void main(String args[])
{
Scanner in = new Scanner(System.in);
System.out.println("Enter 1 to deposit money");
System.out.println("Enter 2 to withdraw money");
System.out.println("Enter 3 to check balance");
System.out.println("Enter 0 to quit");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
double amt = 0.0;
double bal = 5000.0; //Initial balance of account
switch(ch) {
case 0:
System.out.println("Thank you");
break;
case 1:
System.out.print("Enter deposit amount : ");
amt = in.nextDouble();
bal += amt;
System.out.println("Money deposited.");
System.out.print("Total balance = " + bal);
break;
case 2:
System.out.print("Enter withdrawal amount : ");
amt = in.nextDouble();
if(amt > bal) {
System.out.println("Insufficient balance.");
}
else {
bal -= amt;
System.out.println("Money withdrawn.");
System.out.print("Total balance = " + bal);
}
break;
case 3:
System.out.print("Total balance = " + bal);
break;
default:
System.out.println("Invalid request.");
}
}
}
Variable Description Table
Program Explanation
Output
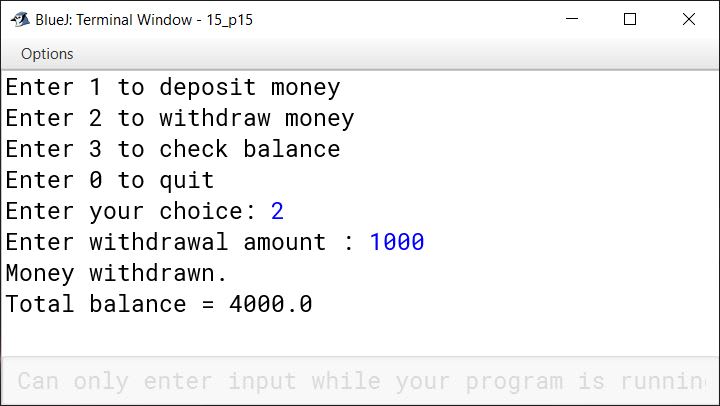
Answered By
16 Likes
Related Questions
Write a menu driven program to calculate:
- Area of a circle = p*r2, where p = (22/7)
- Area of a square = side*side
- Area of a rectangle = length*breadth
Enter 'c' to calculate area of circle, 's' to calculate area of square and 'r' to calculate area of rectangle.
The relative velocity of two trains travelling in opposite directions is calculated by adding their velocities. In case, the trains are travelling in the same direction, the relative velocity is the difference between their velocities. Write a program to input the velocities and length of the trains. Write a menu driven program to calculate the relative velocities and the time taken to cross each other.
In order to purchase an old car, the depreciated value can be calculated as per the tariff given below:
No. of years used Rate of depreciation 1 10% 2 20% 3 30% 4 50% Above 4 years 60% Write a menu driven program to input showroom price and the number of years the car is used ('1' for one year old, '2' for two years old and so on). Calculate the depreciated value. Display the original price of the car, depreciated value and the amount to be paid.
Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h