Computer Applications
In order to purchase an old car, the depreciated value can be calculated as per the tariff given below:
No. of years used | Rate of depreciation |
---|---|
1 | 10% |
2 | 20% |
3 | 30% |
4 | 50% |
Above 4 years | 60% |
Write a menu driven program to input showroom price and the number of years the car is used ('1' for one year old, '2' for two years old and so on). Calculate the depreciated value. Display the original price of the car, depreciated value and the amount to be paid.
Java
Java Conditional Stmts
63 Likes
Answer
import java.util.Scanner;
public class KboatCarValue
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. One year old car");
System.out.println("2. Two year old car");
System.out.println("3. Three year old car");
System.out.println("4. Four year old car");
System.out.println("5. More than four year old car");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
if (choice < 1 || choice > 5) {
System.out.println("Wrong choice! Please select from 1, 2, 3, 4, 5.");
return;
}
System.out.print("Enter showroom price: ");
double price = in.nextDouble();
double depValue = 0.0;
switch(choice) {
case 1:
depValue = 0.1 * price;
break;
case 2:
depValue = 0.2 * price;
break;
case 3:
depValue = 0.3 * price;
break;
case 4:
depValue = 0.5 * price;
break;
case 5:
depValue = 0.6 * price;
break;
default:
System.out.println("Wrong choice! Please select from 1, 2, 3, 4, 5.");
break;
}
double amtPayable = price - depValue;
System.out.println("Original Price = " + price);
System.out.println("Depricated Value = " + depValue);
System.out.println("Amount to be paid = " + amtPayable);
}
}
Variable Description Table
Program Explanation
Output
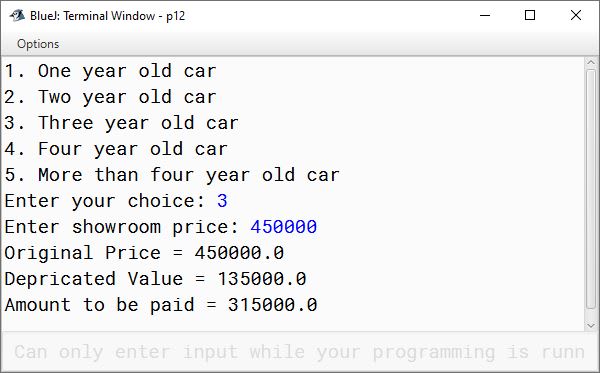
Answered By
26 Likes
Related Questions
Write a menu driven program to calculate:
- Area of a circle = p*r2, where p = (22/7)
- Area of a square = side*side
- Area of a rectangle = length*breadth
Enter 'c' to calculate area of circle, 's' to calculate area of square and 'r' to calculate area of rectangle.
Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h
The relative velocity of two trains travelling in opposite directions is calculated by adding their velocities. In case, the trains are travelling in the same direction, the relative velocity is the difference between their velocities. Write a program to input the velocities and length of the trains. Write a menu driven program to calculate the relative velocities and the time taken to cross each other.
You have a saving account in a bank with some balance amount in your account. Now, you want to perform the following tasks, as per your choice. The tasks are as under.
1. Money Deposited
2. Money Withdrawn
3. Check balance
0. To quitWrite a menu driven program to take input from the user and perform the above tasks. The program checks the balance before withdrawal and finally displays the current balance after transaction. For an incorrect choice, an appropriate message should be displayed.