Computer Applications
Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.
- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h
Java
Java Conditional Stmts
114 Likes
Answer
import java.util.Scanner;
public class KboatMenuVolume
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Volume of Cube");
System.out.println("2. Volume of Sphere");
System.out.println("3. Volume of Cuboid");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
switch(choice) {
case 1:
System.out.print("Enter side of cube: ");
double cs = in.nextDouble();
double cv = Math.pow(cs, 3);
System.out.println("Volume of cube = " + cv);
break;
case 2:
System.out.print("Enter radius of sphere: ");
double r = in.nextDouble();
double sa = (4 / 3.0) * (22 / 7.0) * Math.pow(r, 3);
System.out.println("Volume of sphere = " + sa);
break;
case 3:
System.out.print("Enter length of cuboid: ");
double l = in.nextDouble();
System.out.print("Enter breadth of cuboid: ");
double b = in.nextDouble();
System.out.print("Enter height of cuboid: ");
double h = in.nextDouble();
double vol = l * b * h;
System.out.println("Volume of cuboid = " + vol);
break;
default:
System.out.println("Wrong choice! Please select from 1 or 2 or 3.");
}
}
}
Variable Description Table
Program Explanation
Output
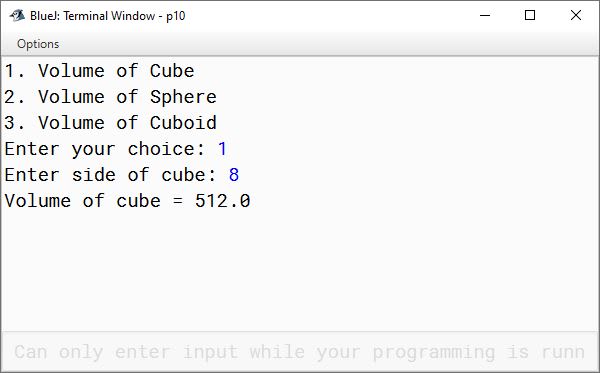
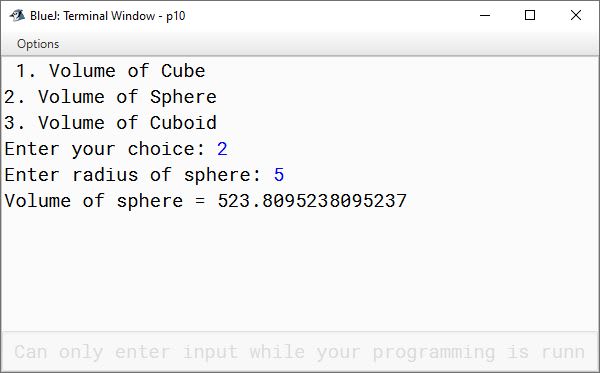
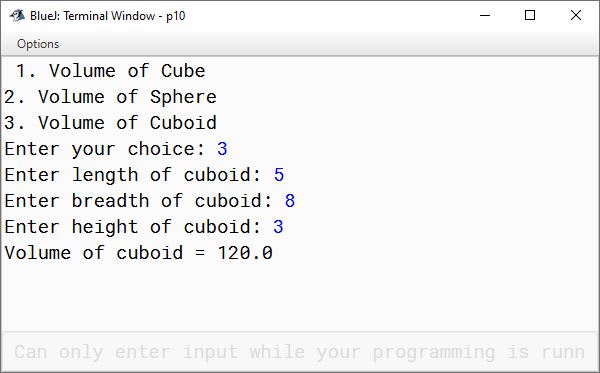
Answered By
28 Likes
Related Questions
Write a program that accepts three numbers from the user and displays them either in "Increasing Order" or in "Decreasing Order" as per the user's choice.
Choice 1: Ascending order
Choice 2: Descending orderSample Inputs: 394, 475, 296
Choice 2
Sample Output
First number : 475
Second number: 394
Third number : 296
The numbers are in decreasing order.Write a menu driven program to calculate:
- Area of a circle = p*r2, where p = (22/7)
- Area of a square = side*side
- Area of a rectangle = length*breadth
Enter 'c' to calculate area of circle, 's' to calculate area of square and 'r' to calculate area of rectangle.
The relative velocity of two trains travelling in opposite directions is calculated by adding their velocities. In case, the trains are travelling in the same direction, the relative velocity is the difference between their velocities. Write a program to input the velocities and length of the trains. Write a menu driven program to calculate the relative velocities and the time taken to cross each other.
In order to purchase an old car, the depreciated value can be calculated as per the tariff given below:
No. of years used Rate of depreciation 1 10% 2 20% 3 30% 4 50% Above 4 years 60% Write a menu driven program to input showroom price and the number of years the car is used ('1' for one year old, '2' for two years old and so on). Calculate the depreciated value. Display the original price of the car, depreciated value and the amount to be paid.