Computer Applications
The relative velocity of two trains travelling in opposite directions is calculated by adding their velocities. In case, the trains are travelling in the same direction, the relative velocity is the difference between their velocities. Write a program to input the velocities and length of the trains. Write a menu driven program to calculate the relative velocities and the time taken to cross each other.
Java
Java Conditional Stmts
74 Likes
Answer
import java.util.Scanner;
public class KboatTrain
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("1. Trains travelling in same direction");
System.out.println("2. Trains travelling in opposite direction");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
System.out.print("Enter first train velocity: ");
double speed1 = in.nextDouble();
System.out.print("Enter first train length: ");
double len1 = in.nextDouble();
System.out.print("Enter second train velocity: ");
double speed2 = in.nextDouble();
System.out.print("Enter second train length: ");
double len2 = in.nextDouble();
double rSpeed = 0.0;
switch(choice) {
case 1:
rSpeed = Math.abs(speed1 - speed2);
break;
case 2:
rSpeed = speed1 + speed2;
break;
default:
System.out.println("Wrong choice! Please select from 1 or 2.");
}
double time = (len1 + len2) / rSpeed;
System.out.println("Relative Velocity = " + rSpeed);
System.out.println("Time taken to cross = " + time);
}
}
Variable Description Table
Program Explanation
Output
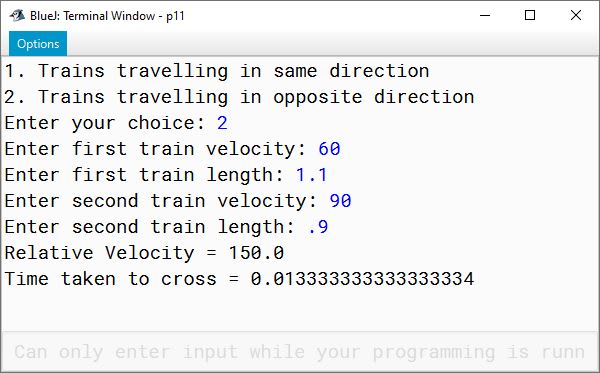
Answered By
24 Likes
Related Questions
Write a menu driven program to calculate:
- Area of a circle = p*r2, where p = (22/7)
- Area of a square = side*side
- Area of a rectangle = length*breadth
Enter 'c' to calculate area of circle, 's' to calculate area of square and 'r' to calculate area of rectangle.
Write a program using switch case to find the volume of a cube, a sphere and a cuboid.
For an incorrect choice, an appropriate error message should be displayed.- Volume of a cube = s * s *s
- Volume of a sphere = (4/3) * π * r * r * r (π = (22/7))
- Volume of a cuboid = l*b*h
In order to purchase an old car, the depreciated value can be calculated as per the tariff given below:
No. of years used Rate of depreciation 1 10% 2 20% 3 30% 4 50% Above 4 years 60% Write a menu driven program to input showroom price and the number of years the car is used ('1' for one year old, '2' for two years old and so on). Calculate the depreciated value. Display the original price of the car, depreciated value and the amount to be paid.
You have a saving account in a bank with some balance amount in your account. Now, you want to perform the following tasks, as per your choice. The tasks are as under.
1. Money Deposited
2. Money Withdrawn
3. Check balance
0. To quitWrite a menu driven program to take input from the user and perform the above tasks. The program checks the balance before withdrawal and finally displays the current balance after transaction. For an incorrect choice, an appropriate message should be displayed.