Computer Applications
Write a program to accept a sentence. Display the sentence in reversing order of its word.
Sample Input: Computer is Fun
Sample Output: Fun is Computer
Java
Java String Handling
171 Likes
Answer
import java.util.Scanner;
public class KboatReverseWords
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str = " " + str;
String word = "";
int len = str.length();
for (int i = len - 1; i >= 0; i--) {
char ch = str.charAt(i);
if (ch == ' ') {
System.out.print(word + " ");
word = "";
}
else {
word = ch + word;
}
}
}
}
Variable Description Table
Program Explanation
Output
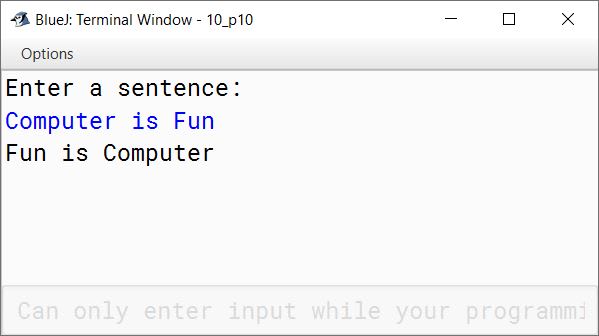
Answered By
60 Likes
Related Questions
Write a program to input a sentence and display the word of the sentence that contains maximum number of vowels.
Sample Input: HAPPY NEW YEAR
Sample Output: The word with maximum number of vowels: YEARWrite a program in Java to enter a sentence. Frame a word by joining all the first characters of each word of the sentence. Display the word.
Sample Input: Vital Information Resource Under Seize
Sample Output: VIRUSWrite a program in Java to enter a sentence. Display the words which are only palindrome.
Sample Input: MOM AND DAD ARE NOT AT HOME
Sample Output: MOM
DADConsider the sentence as given below:
Blue bottle is in Blue bag lying on Blue carpet
Write a program to assign the given sentence to a string variable. Replace the word Blue with Red at all its occurrence. Display the new string as shown below:
Red bottle is in Red bag lying on Red carpet