Computer Applications
Write a program in Java to enter a sentence. Display the words which are only palindrome.
Sample Input: MOM AND DAD ARE NOT AT HOME
Sample Output: MOM
DAD
Java
Java String Handling
148 Likes
Answer
import java.util.Scanner;
public class KboatPalinWords
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str = str + " ";
String word = "";
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ') {
int wordLen = word.length();
boolean isPalin = true;
for (int j = 0; j < wordLen / 2; j++) {
if (word.charAt(j) != word.charAt(wordLen - 1 - j)) {
isPalin = false;
break;
}
}
if (isPalin)
System.out.println(word);
word = "";
}
else {
word += ch;
}
}
}
}
Variable Description Table
Program Explanation
Output
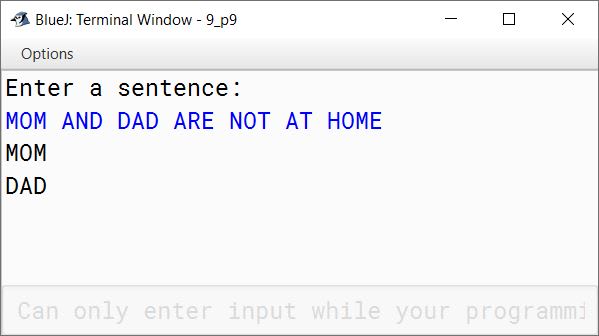
Answered By
46 Likes
Related Questions
Write a program in Java to accept a String in upper case and replace all the vowels present in the String with Asterisk (*) sign.
Sample Input: "TATA STEEL IS IN JAMSHEDPUR"
Sample output: T*T* ST**L *S *N J*MSH*DP*RWrite a program in Java to enter a sentence. Frame a word by joining all the first characters of each word of the sentence. Display the word.
Sample Input: Vital Information Resource Under Seize
Sample Output: VIRUSWrite a program to accept a sentence. Display the sentence in reversing order of its word.
Sample Input: Computer is Fun
Sample Output: Fun is ComputerWrite a program to input a sentence and display the word of the sentence that contains maximum number of vowels.
Sample Input: HAPPY NEW YEAR
Sample Output: The word with maximum number of vowels: YEAR