Computer Applications
Write a program in Java to accept a String in upper case and replace all the vowels present in the String with Asterisk (*) sign.
Sample Input: "TATA STEEL IS IN JAMSHEDPUR"
Sample output: T*T* ST**L *S *N J*MSH*DP*R
Java
Java String Handling
120 Likes
Answer
import java.util.Scanner;
public class KboatVowelReplace
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a string in uppercase:");
String str = in.nextLine();
String newStr = "";
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == 'A' ||
ch == 'E' ||
ch == 'I' ||
ch == 'O' ||
ch == 'U') {
newStr = newStr + '*';
}
else {
newStr = newStr + ch;
}
}
System.out.println(newStr);
}
}
Variable Description Table
Program Explanation
Output
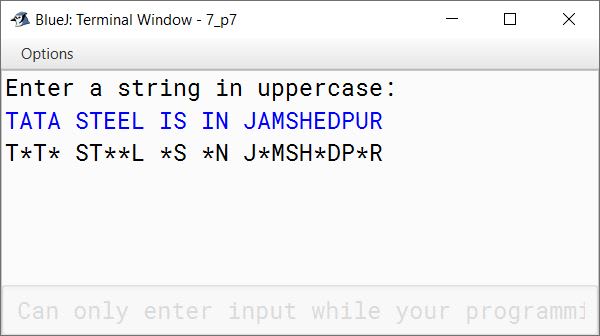
Answered By
41 Likes
Related Questions
Write a program in Java to enter a String/Sentence and display the longest word and the length of the longest word present in the String.
Sample Input: “TATA FOOTBALL ACADEMY WILL PLAY AGAINST MOHAN BAGAN”
Sample Output: The longest word: FOOTBALL: The length of the word: 8Write a program in Java to accept a word and display the ASCII code of each character of the word.
Sample Input: BLUEJ
Sample Output:
ASCII of B = 66
ASCII of L = 76
ASCII of U = 85
ASCII of E = 69
ASCII of J = 74Write a program in Java to enter a sentence. Frame a word by joining all the first characters of each word of the sentence. Display the word.
Sample Input: Vital Information Resource Under Seize
Sample Output: VIRUSWrite a program in Java to enter a sentence. Display the words which are only palindrome.
Sample Input: MOM AND DAD ARE NOT AT HOME
Sample Output: MOM
DAD