Computer Applications
Write a program in Java to enter a sentence. Frame a word by joining all the first characters of each word of the sentence. Display the word.
Sample Input: Vital Information Resource Under Seize
Sample Output: VIRUS
Java
Java String Handling
84 Likes
Answer
import java.util.Scanner;
public class KboatFrameWord
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
String word = "" + str.charAt(0);
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ')
word += str.charAt(i + 1);
}
System.out.println(word);
}
}
Variable Description Table
Program Explanation
Output
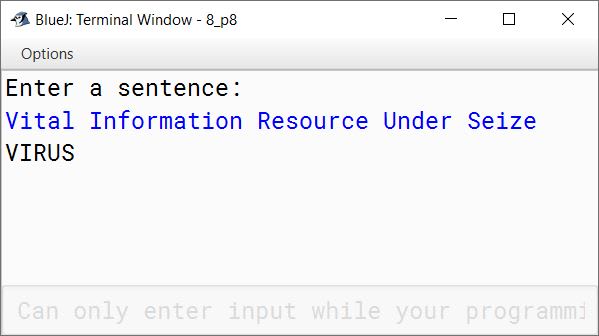
Answered By
31 Likes
Related Questions
Write a program to accept a sentence. Display the sentence in reversing order of its word.
Sample Input: Computer is Fun
Sample Output: Fun is ComputerWrite a program in Java to accept a String in upper case and replace all the vowels present in the String with Asterisk (*) sign.
Sample Input: "TATA STEEL IS IN JAMSHEDPUR"
Sample output: T*T* ST**L *S *N J*MSH*DP*RWrite a program in Java to enter a sentence. Display the words which are only palindrome.
Sample Input: MOM AND DAD ARE NOT AT HOME
Sample Output: MOM
DADWrite a program in Java to accept a word and display the ASCII code of each character of the word.
Sample Input: BLUEJ
Sample Output:
ASCII of B = 66
ASCII of L = 76
ASCII of U = 85
ASCII of E = 69
ASCII of J = 74