Computer Applications
Consider the sentence as given below:
Blue bottle is in Blue bag lying on Blue carpet
Write a program to assign the given sentence to a string variable. Replace the word Blue with Red at all its occurrence. Display the new string as shown below:
Red bottle is in Red bag lying on Red carpet
Java
Java String Handling
54 Likes
Answer
public class KboatStringReplace
{
public static void main(String args[]) {
String str = "Blue bottle is in Blue bag lying on Blue carpet";
str += " ";
String newStr = "";
String word = "";
String target = "Blue";
String newWord = "Red";
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (ch == ' ') {
if (target.equals(word)) {
newStr = newStr + newWord + " ";
}
else {
newStr = newStr + word + " ";
}
word = "";
}
else {
word += ch;
}
}
System.out.println(newStr);
}
}
Variable Description Table
Program Explanation
Output
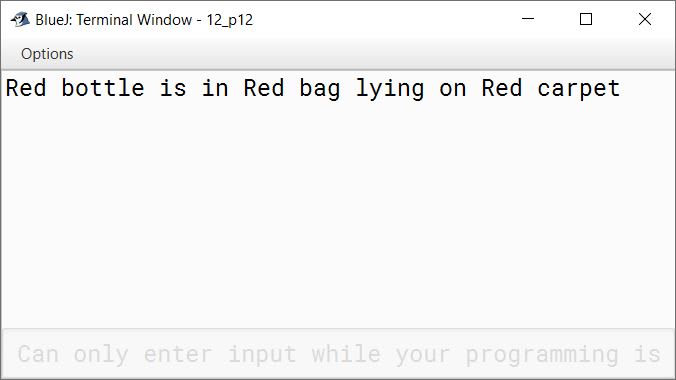
Answered By
17 Likes
Related Questions
Write a program to accept a sentence. Display the sentence in reversing order of its word.
Sample Input: Computer is Fun
Sample Output: Fun is ComputerWrite a program to input a sentence and display the word of the sentence that contains maximum number of vowels.
Sample Input: HAPPY NEW YEAR
Sample Output: The word with maximum number of vowels: YEARWrite a program to accept a word and convert it into lower case, if it is in upper case. Display the new word by replacing only the vowels with the letter following it.
Sample Input: computer
Sample Output: cpmpvtfrWrite a program to input a sentence. Create a new sentence by replacing each consonant with the previous letter. If the previous letter is a vowel then replace it with the next letter (i.e., if the letter is B then replace it with C as the previous letter of B is A). Other characters must remain the same. Display the new sentence.
Sample Input : ICC WORLD CUP
Sample Output : IBB VOQKC BUQ