Computer Applications
Write a program to accept a word and convert it into lower case, if it is in upper case. Display the new word by replacing only the vowels with the letter following it.
Sample Input: computer
Sample Output: cpmpvtfr
Java
Java String Handling
ICSE 2011
167 Likes
Answer
import java.util.Scanner;
public class KboatVowelReplace
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a word: ");
String str = in.nextLine();
str = str.toLowerCase();
String newStr = "";
int len = str.length();
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (str.charAt(i) == 'a' ||
str.charAt(i) == 'e' ||
str.charAt(i) == 'i' ||
str.charAt(i) == 'o' ||
str.charAt(i) == 'u') {
char nextChar = (char)(ch + 1);
newStr = newStr + nextChar;
}
else {
newStr = newStr + ch;
}
}
System.out.println(newStr);
}
}
Variable Description Table
Program Explanation
Output
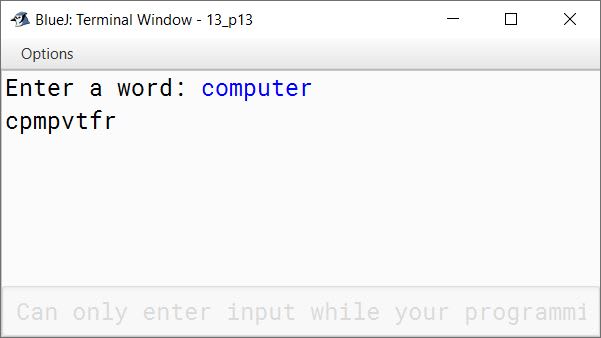
Answered By
52 Likes
Related Questions
Consider the sentence as given below:
Blue bottle is in Blue bag lying on Blue carpet
Write a program to assign the given sentence to a string variable. Replace the word Blue with Red at all its occurrence. Display the new string as shown below:
Red bottle is in Red bag lying on Red carpetWrite a program to input a sentence. Create a new sentence by replacing each consonant with the previous letter. If the previous letter is a vowel then replace it with the next letter (i.e., if the letter is B then replace it with C as the previous letter of B is A). Other characters must remain the same. Display the new sentence.
Sample Input : ICC WORLD CUP
Sample Output : IBB VOQKC BUQWrite a program to input a sentence and display the word of the sentence that contains maximum number of vowels.
Sample Input: HAPPY NEW YEAR
Sample Output: The word with maximum number of vowels: YEARA 'Happy Word' is defined as:
Take a word and calculate the word’s value based on position of the letters in English alphabet. On the basis of word’s value, find the sum of the squares of its digits. Repeat the process with the resultant number until the number equals 1 (one). If the number ends with 1 then the word is called a 'Happy Word'.
Write a program to input a word and check whether it a ‘Happy Word’ or not. The program displays a message accordingly.
Sample Input: VAT
Place value of V = 22, A= 1, T = 20
[Hint: A = 1, B = 2, ----------, Z = 26]Solution:
22120 ⇒ 22 + 22 + 12 + 22 + 02 = 13
⇒ 12 + 32 = 10
⇒ 12 + 02 = 1Sample Output: A Happy Word