Computer Science
Write a program in Java to accept a string. Count and display the frequency of each character present in the string. The character with multiple frequencies should be displayed only once.
Sample Input:
golden jubilee
Sample Output:
Alphabet | g | o | l | d | e | n | j | u | b | i |
Frequency | 1 | 1 | 2 | 1 | 3 | 1 | 1 | 1 | 1 | 1 |
Java
Java String Handling
26 Likes
Answer
import java.util.Scanner;
public class KboatStringFreq
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a string:");
String str = in.nextLine();
int len = str.length();
char seenArr[] = new char[len];
int freqArr[] = new int[len];
int idx = 0;
for (int i = 0; i < len; i++) {
char ch = str.charAt(i);
if (Character.isWhitespace(ch)) {
continue;
}
boolean seen = false;
for (int j = 0; j < idx; j++) {
if (ch == seenArr[j]) {
seen = true;
break;
}
}
if (seen) {
continue;
}
int f = 1;
for (int k = i + 1; k < len; k++) {
if (ch == str.charAt(k)) {
f++;
}
}
seenArr[idx] = ch;
freqArr[idx] = f;
idx++;
}
for (int i = 0; i < idx; i++) {
System.out.print(seenArr[i] + " ");
}
System.out.println();
for (int i = 0; i < idx; i++) {
System.out.print(freqArr[i] + " ");
}
}
}
Output
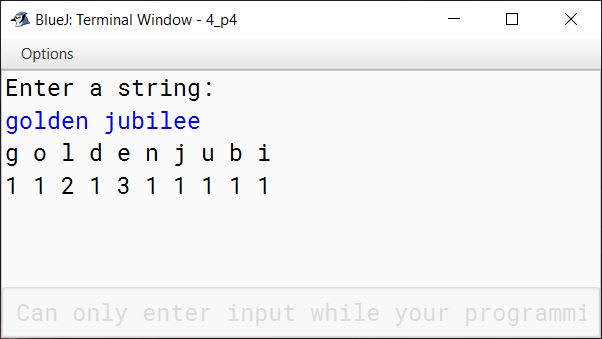
Answered By
8 Likes
Related Questions
Write a program in Java to enter a string in a mixed case. Arrange all the letters of string such that all the lower case characters are followed by the upper case characters.
Sample Input:
Computer ScienceSample Output:
omputercienceCSWrite a program in Java to accept two strings. Display the new string by taking each character of the first string from left to right and of the second string from right to left. The letters should be taken alternatively from each string. Assume that the length of both the strings are same.
Sample Input:
String 1: HISTORY
String 2: SCIENCESample Output:
HEICSNTEOIRCYSWrite a program in Java to accept a string. Arrange all the letters of the string in an alphabetical order. Now, insert the missing letters in the sorted string to complete all the letters between first and last characters of the string.
Sample Input:
computerAlphabetical order:
cemoprtuSample Output:
cdefghijklmnopqrstuWrite a program in Java to accept a string and display the new string after reversing the characters of each word.
Sample Input:
Understanding Computer ScienceSample output:
gnidnatsrednU retupmoC ecneicS