Computer Science
Write a program in Java to accept a string and display the new string after reversing the characters of each word.
Sample Input:
Understanding Computer Science
Sample output:
gnidnatsrednU retupmoC ecneicS
Java
Java String Handling
48 Likes
Answer
import java.util.*;
public class KboatReverse
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a string:");
String str = in.nextLine();
int len = str.length();
String revStr = ""; //Empty String
StringTokenizer st = new StringTokenizer(str);
while (st.hasMoreTokens()) {
String word = st.nextToken();
int wordLen = word.length();
for (int i = wordLen - 1; i >= 0; i--) {
revStr += word.charAt(i);
}
revStr += " ";
}
System.out.println("String with words reversed:");
System.out.println(revStr);
}
}
Output
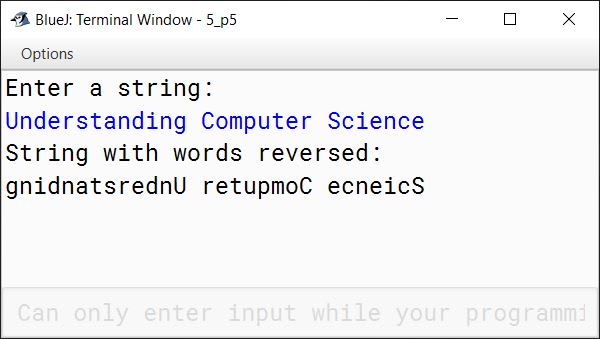
Answered By
17 Likes
Related Questions
Write a program in Java to accept a string. Count and display the frequency of each character present in the string. The character with multiple frequencies should be displayed only once.
Sample Input:
golden jubileeSample Output:
Alphabet g o l d e n j u b i Frequency 1 1 2 1 3 1 1 1 1 1 Write a program in Java to accept a string. Arrange all the letters of the string in an alphabetical order. Now, insert the missing letters in the sorted string to complete all the letters between first and last characters of the string.
Sample Input:
computerAlphabetical order:
cemoprtuSample Output:
cdefghijklmnopqrstuWrite a program in Java to accept a four-letter word. Display all the probable four letter combinations such that no letter should be repeated in the output within each combination.
Sample Input:
PARKSample Output:
PAKR, PKAR, PRAK, APRK, ARPK, AKPR, and so on.Write a program in Java to accept two strings. Display the new string by taking each character of the first string from left to right and of the second string from right to left. The letters should be taken alternatively from each string. Assume that the length of both the strings are same.
Sample Input:
String 1: HISTORY
String 2: SCIENCESample Output:
HEICSNTEOIRCYS