Computer Science
Write a program in Java to accept a string. Arrange all the letters of the string in an alphabetical order. Now, insert the missing letters in the sorted string to complete all the letters between first and last characters of the string.
Sample Input:
computer
Alphabetical order:
cemoprtu
Sample Output:
cdefghijklmnopqrstu
Java
Java String Handling
85 Likes
Answer
import java.util.Scanner;
public class KboatStringSort
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a string:");
String str = in.nextLine();
str = str.toLowerCase();
int len = str.length();
String sortedStr = ""; //Empty String
for (char ch = 'a'; ch <= 'z'; ch++) {
for (int i = 0; i < len; i++) {
char strCh = str.charAt(i);
if (ch == strCh) {
sortedStr += strCh;
}
}
}
System.out.println("Alphabetical order:");
System.out.println(sortedStr);
String filledStr = ""; //Empty String
for (int i = 0; i < len - 1; i++) {
char strCh = sortedStr.charAt(i);
filledStr += strCh;
for (int j = strCh + 1; j < sortedStr.charAt(i+1); j++) {
filledStr += (char)j;
}
}
filledStr += sortedStr.charAt(len - 1);
System.out.println("Filled String:");
System.out.println(filledStr);
}
}
Output
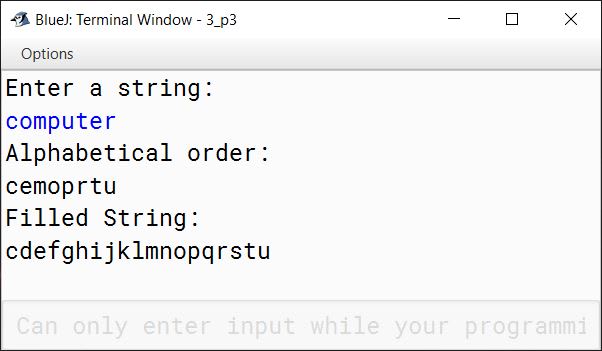
Answered By
31 Likes
Related Questions
A string is given as:
Purity of Mind is EssentialWrite a program in Java to enter the string. Count and display:
- The character with lowest ASCII code in lower case
- The character with highest ASCII code in lower case
- The character with lowest ASCII code in upper case
- The character with highest ASCII code in upper case
Sample Output:
The character with lowest ASCII code in lower case: a
The character with highest ASCII code in lower case: y
The character with lowest ASCII code in upper case: E
The character with highest ASCII code in upper case: PWrite a program in Java to enter a string in a mixed case. Arrange all the letters of string such that all the lower case characters are followed by the upper case characters.
Sample Input:
Computer ScienceSample Output:
omputercienceCSWrite a program in Java to accept a string. Count and display the frequency of each character present in the string. The character with multiple frequencies should be displayed only once.
Sample Input:
golden jubileeSample Output:
Alphabet g o l d e n j u b i Frequency 1 1 2 1 3 1 1 1 1 1 Write a program in Java to accept a string and display the new string after reversing the characters of each word.
Sample Input:
Understanding Computer ScienceSample output:
gnidnatsrednU retupmoC ecneicS