Computer Science
Write a program in Java to accept two strings. Display the new string by taking each character of the first string from left to right and of the second string from right to left. The letters should be taken alternatively from each string. Assume that the length of both the strings are same.
Sample Input:
String 1: HISTORY
String 2: SCIENCE
Sample Output:
HEICSNTEOIRCYS
Java
Java String Handling
26 Likes
Answer
import java.util.Scanner;
public class KboatString
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first String: ");
String s1 = in.nextLine();
System.out.print("Enter second String: ");
String s2 = in.nextLine();
String newStr = "";
int len = s1.length();
for (int i = 0; i < len; i++) {
char ch1 = s1.charAt(i);
char ch2 = s2.charAt(len - 1 - i);
newStr = newStr + ch1 + ch2;
}
System.out.println(newStr);
}
}
Output
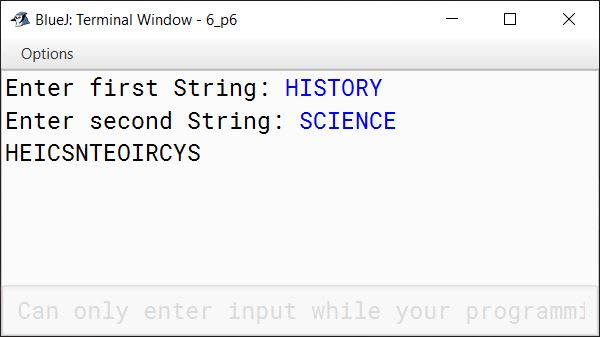
Answered By
10 Likes
Related Questions
Write a program in Java to accept a string. Count and display the frequency of each character present in the string. The character with multiple frequencies should be displayed only once.
Sample Input:
golden jubileeSample Output:
Alphabet g o l d e n j u b i Frequency 1 1 2 1 3 1 1 1 1 1 Write a program in Java to accept a string and display the new string after reversing the characters of each word.
Sample Input:
Understanding Computer ScienceSample output:
gnidnatsrednU retupmoC ecneicSWrite a program in Java to accept a four-letter word. Display all the probable four letter combinations such that no letter should be repeated in the output within each combination.
Sample Input:
PARKSample Output:
PAKR, PKAR, PRAK, APRK, ARPK, AKPR, and so on.While typing, a typist has created two or more consecutive blank spaces between the words of a sentence. Write a program in Java to eliminate multiple blanks between the words by a single blank.
Sample Input:
Indian Cricket team tour to AustraliaSample Output:
Indian Cricket team tour to Australia