Computer Applications
Write a menu driven program to display the following menu:
Conversion Table
============
- Milliseconds to Seconds
- Milliseconds to Minutes
- Seconds to Milliseconds
- Seconds to Minutes
- Minutes to Milliseconds
- Minutes to Seconds
For an incorrect choice, display an appropriate error message.
Hint: 1 second = 1000 milliseconds
Java
Java Conditional Stmts
18 Likes
Answer
import java.util.Scanner;
public class KboatTimeConversion
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Conversion Table");
System.out.println("================");
System.out.println("1. Milliseconds to Seconds");
System.out.println("2. Milliseconds to Minutes");
System.out.println("3. Seconds to Milliseconds");
System.out.println("4. Seconds to Minutes");
System.out.println("5. Minutes to Milliseconds");
System.out.println("6. Minutes to Seconds");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
double ip = 0, op = 0;
switch (choice) {
case 1:
System.out.print("Enter Milliseconds: ");
ip = in.nextDouble();
op = ip / 1000;
System.out.println("Seconds = " + op);
break;
case 2:
System.out.print("Enter Milliseconds: ");
ip = in.nextDouble();
op = ip / 1000 / 60;
System.out.println("Minutes = " + op);
break;
case 3:
System.out.print("Enter Seconds: ");
ip = in.nextDouble();
op = ip * 1000;
System.out.println("Milliseconds = " + op);
break;
case 4:
System.out.print("Enter Seconds: ");
ip = in.nextDouble();
op = ip / 60;
System.out.println("Minutes = " + op);
break;
case 5:
System.out.print("Enter Minutes: ");
ip = in.nextDouble();
op = ip * 60 * 1000;
System.out.println("Milliseconds = " + op);
break;
case 6:
System.out.print("Enter Minutes: ");
ip = in.nextDouble();
op = ip * 60;
System.out.println("Seconds = " + op);
break;
default:
System.out.println("Incorrect choice");
}
}
}
Output
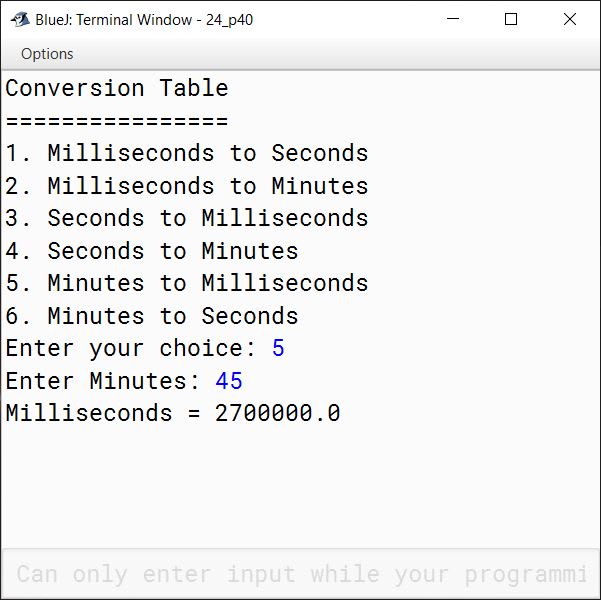
Answered By
7 Likes
Related Questions
A box of cookies can hold 24 cookies, and a container can hold 75 boxes of cookies. Write a program that prompts the user to enter the total number of cookies, the number of cookies in each box, and the number of cookies boxes in a container. The program then outputs the number of boxes and the number of containers required to ship the cookies.
Using switch case statement in Java, create a program to convert rupee into dollar and dollar into rupee according to the user's choice. Assume conversion price: 1 Dollar = Rs.77.
A new taxi service based on electric vehicles is offering services within a metro city. Following is the fare chart including the type of taxi used to commute and price per kilometer. Create a program to calculate total fare depending on the distance travelled in kilometers.
Type Fare Micro 10.05/Km Macro 15.05/Km Shared 7.05/Km Using the switch statement, write a menu driven program:
- To check and display whether a number input by the user is a composite number or not.
A number is said to be composite, if it has one or more than one factors excluding 1 and the number itself.
Example: 4, 6, 8, 9… - To find the smallest digit of an integer that is input:
Sample input: 6524
Sample output: Smallest digit is 2
For an incorrect choice, an appropriate error message should be displayed.
- To check and display whether a number input by the user is a composite number or not.