Computer Applications
Using switch case statement in Java, create a program to convert rupee into dollar and dollar into rupee according to the user's choice. Assume conversion price: 1 Dollar = Rs.77.
Java
Java Conditional Stmts
10 Likes
Answer
import java.util.Scanner;
public class KboatRupeeDollar
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for Rupee to Dollar conversion: ");
System.out.println("Type 2 for Dollar to Rupee conversion: ");
System.out.print("Enter your choice: ");
int choice = in.nextInt();
switch (choice) {
case 1:
System.out.print("Enter rupee amount: ");
double r1 = in.nextDouble();
double d1 = r1 / 77;
System.out.println(r1 + " rupees => " + d1 + " dollars");
break;
case 2:
System.out.print("Enter dollar amount: ");
double d2 = in.nextDouble();
double r2 = d2 * 77;
System.out.println(d2 + " dollars => " + r2 + " rupees");
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Output
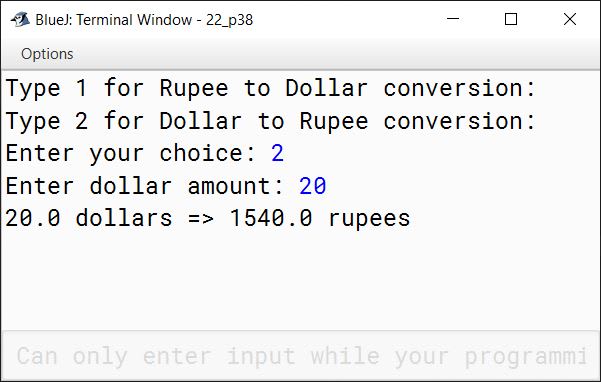
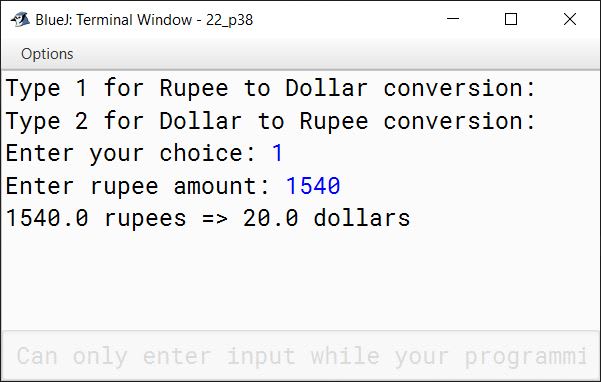
Answered By
3 Likes
Related Questions
A cloth showroom has announced the following festival discounts on the purchase of items based on the total cost of the items purchased:
Total Cost Discount Rate Less than Rs. 2000 5% Rs. 2000 to less than Rs. 5000 25% Rs. 5000 to less than Rs. 10,000 35% Rs. 10,000 and above 50% Write a program to input the total cost and to compute and display the amount to be paid by the customer availing the the discount.
A cloth manufacturing company offers discounts to the dealers and retailers. The discount is computed using the length of the cloth as per the following table:
Length of cloth Dealer's Discount Retailer's Discount Up to 1000 meters 20% 15% Above 1000 meters but less than 2000 meters 25% 20% More than 2000 meters 35% 25% Write a program in Java to input the length of the cloth and the total amount of purchase. The program should display a menu to accept type of customer — Dealer (D) or Retailer (R) and print the amount to be paid. Display a suitable error message for a wrong choice.
A box of cookies can hold 24 cookies, and a container can hold 75 boxes of cookies. Write a program that prompts the user to enter the total number of cookies, the number of cookies in each box, and the number of cookies boxes in a container. The program then outputs the number of boxes and the number of containers required to ship the cookies.
Write a menu driven program to display the following menu:
Conversion Table
============- Milliseconds to Seconds
- Milliseconds to Minutes
- Seconds to Milliseconds
- Seconds to Minutes
- Minutes to Milliseconds
- Minutes to Seconds
For an incorrect choice, display an appropriate error message.
Hint: 1 second = 1000 milliseconds