Computer Applications
A box of cookies can hold 24 cookies, and a container can hold 75 boxes of cookies. Write a program that prompts the user to enter the total number of cookies, the number of cookies in each box, and the number of cookies boxes in a container. The program then outputs the number of boxes and the number of containers required to ship the cookies.
Java
Java Conditional Stmts
36 Likes
Answer
import java.util.Scanner;
public class KboatCookies
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter total no. of cookies: ");
int cookieCount = in.nextInt();
System.out.print("Enter no. of cookies per box: ");
int cookiePerBox = in.nextInt();
System.out.print("Enter no. of boxes per container: ");
int boxPerContainer = in.nextInt();
int numBox = cookieCount / cookiePerBox;
if (cookieCount % cookiePerBox != 0)
numBox++;
int numContainer = numBox / boxPerContainer;
if (cookieCount % cookiePerBox != 0)
numContainer++;
System.out.println("No. of boxes = " + numBox);
System.out.println("No. of containers = " + numContainer);
}
}
Output
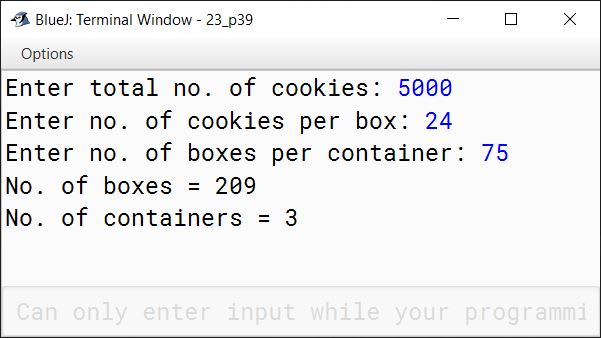
Answered By
20 Likes
Related Questions
Using switch case statement in Java, create a program to convert rupee into dollar and dollar into rupee according to the user's choice. Assume conversion price: 1 Dollar = Rs.77.
Write a menu driven program to display the following menu:
Conversion Table
============- Milliseconds to Seconds
- Milliseconds to Minutes
- Seconds to Milliseconds
- Seconds to Minutes
- Minutes to Milliseconds
- Minutes to Seconds
For an incorrect choice, display an appropriate error message.
Hint: 1 second = 1000 milliseconds
Using the switch statement, write a menu driven program:
- To check and display whether a number input by the user is a composite number or not.
A number is said to be composite, if it has one or more than one factors excluding 1 and the number itself.
Example: 4, 6, 8, 9… - To find the smallest digit of an integer that is input:
Sample input: 6524
Sample output: Smallest digit is 2
For an incorrect choice, an appropriate error message should be displayed.
- To check and display whether a number input by the user is a composite number or not.
A cloth manufacturing company offers discounts to the dealers and retailers. The discount is computed using the length of the cloth as per the following table:
Length of cloth Dealer's Discount Retailer's Discount Up to 1000 meters 20% 15% Above 1000 meters but less than 2000 meters 25% 20% More than 2000 meters 35% 25% Write a program in Java to input the length of the cloth and the total amount of purchase. The program should display a menu to accept type of customer — Dealer (D) or Retailer (R) and print the amount to be paid. Display a suitable error message for a wrong choice.