Computer Applications
Using the switch statement, write a menu driven program:
- To check and display whether a number input by the user is a composite number or not.
A number is said to be composite, if it has one or more than one factors excluding 1 and the number itself.
Example: 4, 6, 8, 9… - To find the smallest digit of an integer that is input:
Sample input: 6524
Sample output: Smallest digit is 2
For an incorrect choice, an appropriate error message should be displayed.
Java
Java Conditional Stmts
ICSE 2013
162 Likes
Answer
import java.util.Scanner;
public class KboatNumber
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for Composite Number");
System.out.println("Type 2 for Smallest Digit");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
System.out.print("Enter Number: ");
int n = in.nextInt();
int c = 0;
for (int i = 1; i <= n; i++) {
if (n % i == 0)
c++;
}
if (c > 2)
System.out.println("Composite Number");
else
System.out.println("Not a Composite Number");
break;
case 2:
System.out.print("Enter Number: ");
int num = in.nextInt();
int s = 10;
while (num != 0) {
int d = num % 10;
if (d < s)
s = d;
num /= 10;
}
System.out.println("Smallest digit is " + s);
break;
default:
System.out.println("Wrong choice");
}
}
}
Output
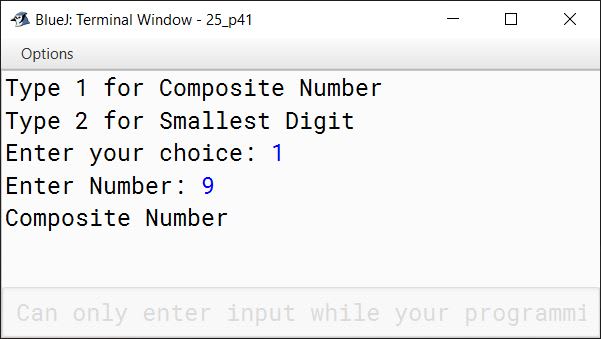
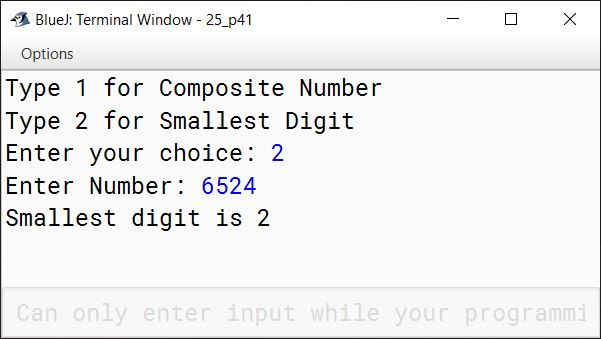
Answered By
57 Likes
Related Questions
The City Library charges late fine from members if the books were not returned on time as per the following table:
Number of days late Magazines fine per day Text books fine per day Up to 5 days Rs. 1 Rs. 2 6 to 10 days Rs. 2 Rs. 3 11 to 15 days Rs. 3 Rs. 4 16 to 20 days Rs. 5 Rs. 6 More than 20 days Rs. 6 Rs. 7 Using the switch statement, write a program in Java to input name of person, number of days late and type of book — 'M' for Magazine and 'T' for Text book. Compute the total fine and display it along with the name of the person.
A box of cookies can hold 24 cookies, and a container can hold 75 boxes of cookies. Write a program that prompts the user to enter the total number of cookies, the number of cookies in each box, and the number of cookies boxes in a container. The program then outputs the number of boxes and the number of containers required to ship the cookies.
Write a menu driven program to display the following menu:
Conversion Table
============- Milliseconds to Seconds
- Milliseconds to Minutes
- Seconds to Milliseconds
- Seconds to Minutes
- Minutes to Milliseconds
- Minutes to Seconds
For an incorrect choice, display an appropriate error message.
Hint: 1 second = 1000 milliseconds
A new taxi service based on electric vehicles is offering services within a metro city. Following is the fare chart including the type of taxi used to commute and price per kilometer. Create a program to calculate total fare depending on the distance travelled in kilometers.
Type Fare Micro 10.05/Km Macro 15.05/Km Shared 7.05/Km