Computer Applications
Define a class Student with the following specifications:
Class name : Student
Data Members | Purpose |
---|---|
String name | To store the name of the student |
int eng | To store marks in English |
int hn | To store marks in Hindi |
int mts | To store marks in Maths |
double total | To store total marks |
double avg | To store average marks |
Member Methods | Purpose |
---|---|
void accept() | To input marks in English, Hindi and Maths |
void compute() | To calculate total marks and average of 3 subjects |
void display() | To show all the details viz. name, marks, total and average |
Write a program to create an object and invoke the above methods.
Java
Java Classes
47 Likes
Answer
import java.util.Scanner;
public class Student
{
private String name;
private int eng;
private int hn;
private int mts;
private double total;
private double avg;
public void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter student name: ");
name = in.nextLine();
System.out.print("Enter marks in English: ");
eng = in.nextInt();
System.out.print("Enter marks in Hindi: ");
hn = in.nextInt();
System.out.print("Enter marks in Maths: ");
mts = in.nextInt();
}
public void compute() {
total = eng + hn + mts;
avg = total / 3.0;
}
public void display() {
System.out.println("Name: " + name);
System.out.println("Marks in English: " + eng);
System.out.println("Marks in Hindi: " + hn);
System.out.println("Marks in Maths: " + mts);
System.out.println("Total Marks: " + total);
System.out.println("Average Marks: " + avg);
}
public static void main(String args[]) {
Student obj = new Student();
obj.accept();
obj.compute();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
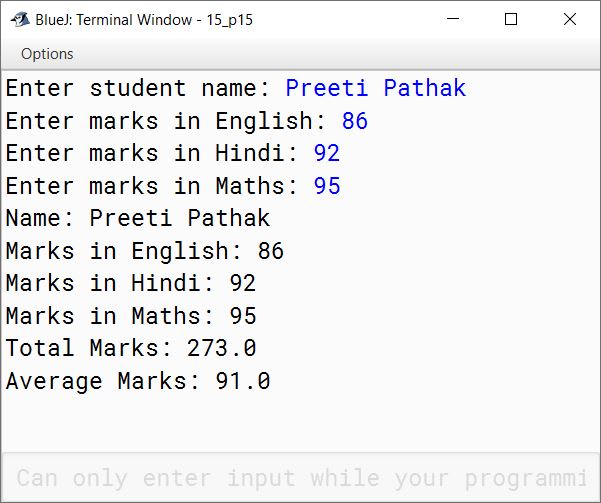
Answered By
17 Likes
Related Questions
Write a program by using class with the following specifications:
Class name — Characters
Data Members:
- String str — To store the string
Member Methods:
- void input (String st) — to assign st to str
- void check_print() — to check and print the following:
(i) number of letters
(ii) number of digits
(iii) number of uppercase characters
(iv) number of lowercase characters
(v) number of special characters
Design a class RailwayTicket with following description:
Class name : RailwayTicket
Data Members Purpose String name To store the name of the customer String coach To store the type of coach customer wants to travel long mob no To store customer's mobile number int amt To store basic amount of ticket int totalamt To store the amount to be paid after updating the original amount Member Methods Purpose void accept() To take input for name, coach, mobile number and amount void update() To update the amount as per the coach selected (extra amount to be added in the amount as per the table below) void display() To display all details of a customer such as name, coach, total amount and mobile number Type of Coaches Amount First_AC ₹700 Second_AC ₹500 Third_AC ₹250 Sleeper None Write a main method to create an object of the class and call the above member methods.
A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.
Define a class called ParkingLot with the following description:
Class name : ParkingLot
Data Members Purpose int vno To store the vehicle number int hours To store the number of hours the vehicle is parked in the parking lot double bill To store the bill amount Member Methods Purpose void input( ) To input the vno and hours void calculate( ) To compute the parking charge at the rate ₹3 for the first hour or the part thereof and ₹1.50 for each additional hour or part thereof. void display() To display the detail Write a main method to create an object of the class and call the above methods.