Computer Applications
A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.
Java
Java Classes
23 Likes
Answer
import java.util.Scanner;
public class Stock
{
private String title;
private String author;
private String pub;
private int noc;
public void getdata() {
Scanner in = new Scanner(System.in);
System.out.print("Enter book title: ");
title = in.nextLine();
System.out.print("Enter book author: ");
author = in.nextLine();
System.out.print("Enter book publisher: ");
pub = in.nextLine();
System.out.print("Enter no. of copies: ");
noc = in.nextInt();
}
public void purchase(String t, String a, String p, int n) {
if (title.equalsIgnoreCase(t) &&
author.equalsIgnoreCase(a) &&
pub.equalsIgnoreCase(p)) {
if (noc > n) {
noc -= n;
System.out.println("Updated noc = " + noc);
}
else {
System.out.println("Stock is under flowing");
}
}
else {
System.out.println("Book is not available");
}
}
public static void main(String args[]) {
Stock obj = new Stock();
obj.getdata();
obj.purchase("wings of fire", "APJ Abdul Kalam",
"universities press", 10);
obj.purchase("Ignited Minds", "APJ Abdul Kalam",
"Penguin", 5);
obj.purchase("wings of fire", "APJ Abdul Kalam",
"universities press", 20);
}
}
Variable Description Table
Program Explanation
Output
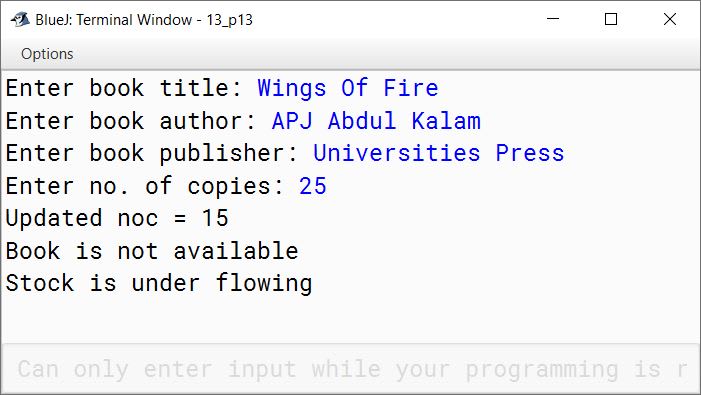
Answered By
6 Likes
Related Questions
Write a program using a class with the following specifications:
Class name: Caseconvert
Data Members Purpose String str To store the string Member Methods Purpose void getstr() to accept a string void convert() to obtain a string after converting each upper case letter into lower case and vice versa void display() to print the converted string Write a program by using a class with the following specifications:
Class name: Vowel
Data Members Purpose String s To store the string int c To count vowels Member Methods Purpose void getstr() to accept a string void getvowel() to count the number of vowels void display() to print the number of vowels Write a program by using class with the following specifications:
Class name — Characters
Data Members:
- String str — To store the string
Member Methods:
- void input (String st) — to assign st to str
- void check_print() — to check and print the following:
(i) number of letters
(ii) number of digits
(iii) number of uppercase characters
(iv) number of lowercase characters
(v) number of special characters
Define a class Student with the following specifications:
Class name : Student
Data Members Purpose String name To store the name of the student int eng To store marks in English int hn To store marks in Hindi int mts To store marks in Maths double total To store total marks double avg To store average marks Member Methods Purpose void accept() To input marks in English, Hindi and Maths void compute() To calculate total marks and average of 3 subjects void display() To show all the details viz. name, marks, total and average Write a program to create an object and invoke the above methods.