Computer Applications
Write a program by using class with the following specifications:
Class name — Characters
Data Members:
- String str — To store the string
Member Methods:
- void input (String st) — to assign st to str
- void check_print() — to check and print the following:
(i) number of letters
(ii) number of digits
(iii) number of uppercase characters
(iv) number of lowercase characters
(v) number of special characters
Java
Java Classes
25 Likes
Answer
import java.util.Scanner;
public class Characters
{
private String str;
public void input(String st) {
str = st;
}
public void check_print() {
int cLetters = 0, cDigits = 0, cUpper = 0, cLower = 0,
cSpecial = 0;
for (int i = 0; i < str.length(); i++) {
char ch = str.charAt(i);
if (ch >= 'A' && ch <= 'Z') {
cLetters++;
cUpper++;
}
else if (ch >= 'a' && ch <= 'z') {
cLetters++;
cLower++;
}
else if (ch >= '0' && ch <= '9') {
cDigits++;
}
else if (!Character.isWhitespace(ch)) {
cSpecial++;
}
}
System.out.println("Number of Letters: " + cLetters);
System.out.println("Number of Digits: " + cDigits);
System.out.println("Number of Upppercase Characters: "
+ cUpper);
System.out.println("Number of Lowercase Characters: "
+ cLower);
System.out.println("Number of Special Characters: "
+ cSpecial);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the string: ");
String s = in.nextLine();
Characters obj = new Characters();
obj.input(s);
obj.check_print();
}
}
Variable Description Table
Program Explanation
Output
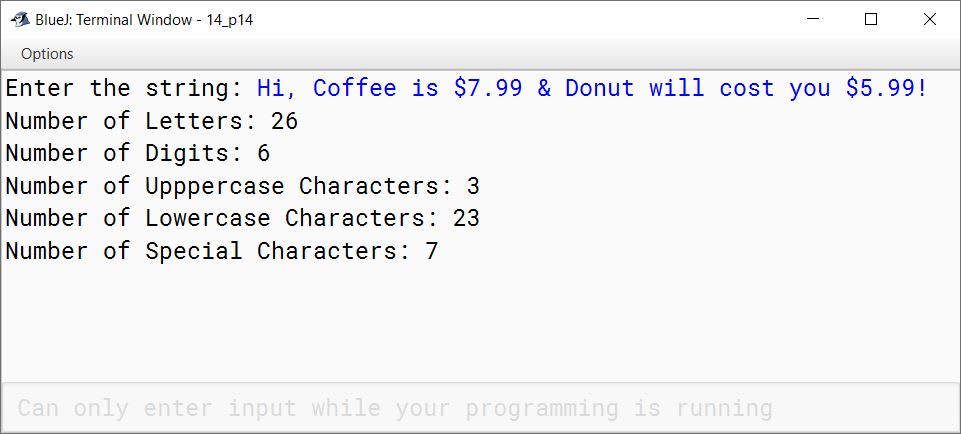
Answered By
10 Likes
Related Questions
Write a program by using a class with the following specifications:
Class name: Vowel
Data Members Purpose String s To store the string int c To count vowels Member Methods Purpose void getstr() to accept a string void getvowel() to count the number of vowels void display() to print the number of vowels A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.
Define a class Student with the following specifications:
Class name : Student
Data Members Purpose String name To store the name of the student int eng To store marks in English int hn To store marks in Hindi int mts To store marks in Maths double total To store total marks double avg To store average marks Member Methods Purpose void accept() To input marks in English, Hindi and Maths void compute() To calculate total marks and average of 3 subjects void display() To show all the details viz. name, marks, total and average Write a program to create an object and invoke the above methods.
Define a class called ParkingLot with the following description:
Class name : ParkingLot
Data Members Purpose int vno To store the vehicle number int hours To store the number of hours the vehicle is parked in the parking lot double bill To store the bill amount Member Methods Purpose void input( ) To input the vno and hours void calculate( ) To compute the parking charge at the rate ₹3 for the first hour or the part thereof and ₹1.50 for each additional hour or part thereof. void display() To display the detail Write a main method to create an object of the class and call the above methods.