Computer Applications
Design a class RailwayTicket with following description:
Class name : RailwayTicket
Data Members | Purpose |
---|---|
String name | To store the name of the customer |
String coach | To store the type of coach customer wants to travel |
long mob no | To store customer's mobile number |
int amt | To store basic amount of ticket |
int totalamt | To store the amount to be paid after updating the original amount |
Member Methods | Purpose |
---|---|
void accept() | To take input for name, coach, mobile number and amount |
void update() | To update the amount as per the coach selected (extra amount to be added in the amount as per the table below) |
void display() | To display all details of a customer such as name, coach, total amount and mobile number |
Type of Coaches | Amount |
---|---|
First_AC | ₹700 |
Second_AC | ₹500 |
Third_AC | ₹250 |
Sleeper | None |
Write a main method to create an object of the class and call the above member methods.
Java
Java Classes
ICSE 2018
155 Likes
Answer
import java.util.Scanner;
public class RailwayTicket
{
private String name;
private String coach;
private long mobno;
private int amt;
private int totalamt;
private void accept() {
Scanner in = new Scanner(System.in);
System.out.print("Enter name: ");
name = in.nextLine();
System.out.print("Enter coach: ");
coach = in.nextLine();
System.out.print("Enter mobile no: ");
mobno = in.nextLong();
System.out.print("Enter amount: ");
amt = in.nextInt();
}
private void update() {
if(coach.equalsIgnoreCase("First_AC"))
totalamt = amt + 700;
else if(coach.equalsIgnoreCase("Second_AC"))
totalamt = amt + 500;
else if(coach.equalsIgnoreCase("Third_AC"))
totalamt = amt + 250;
else if(coach.equalsIgnoreCase("Sleeper"))
totalamt = amt;
}
private void display() {
System.out.println("Name: " + name);
System.out.println("Coach: " + coach);
System.out.println("Total Amount: " + totalamt);
System.out.println("Mobile number: " + mobno);
}
public static void main(String args[]) {
RailwayTicket obj = new RailwayTicket();
obj.accept();
obj.update();
obj.display();
}
}
Variable Description Table
Program Explanation
Output
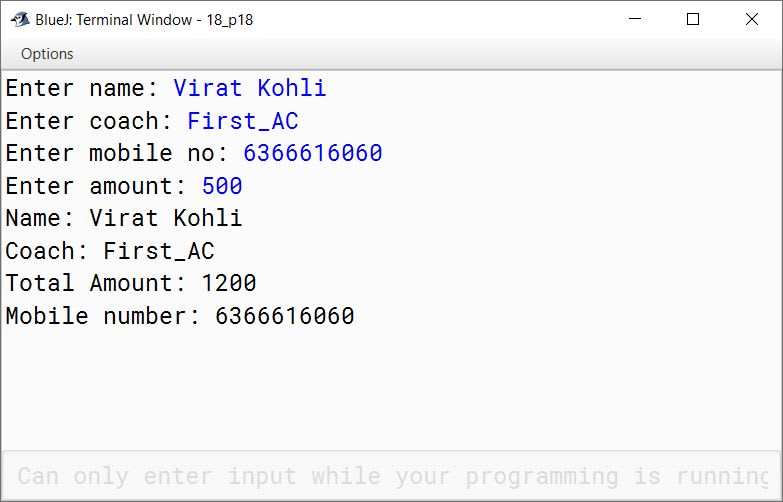
Answered By
78 Likes
Related Questions
A bookseller maintains record of books belonging to the various publishers. He uses a class with the specifications given below:
Class name — Stock
Data Members:
- String title — Contains title of the book
- String author — Contains author name
- String pub — Contains publisher's name
- int noc — Number of copies
Member Methods:
- void getdata() — To accept title, author, publisher's name and the number of copies.
- void purchase(int t, String a, String p, int n) — To check the existence of the book in the stock by comparing total, author's and publisher's name. Also check whether noc >n or not. If yes, maintain the balance as noc-n, otherwise display book is not available or stock is under flowing.
Write a program to perform the task given above.
Define a class called ParkingLot with the following description:
Class name : ParkingLot
Data Members Purpose int vno To store the vehicle number int hours To store the number of hours the vehicle is parked in the parking lot double bill To store the bill amount Member Methods Purpose void input( ) To input the vno and hours void calculate( ) To compute the parking charge at the rate ₹3 for the first hour or the part thereof and ₹1.50 for each additional hour or part thereof. void display() To display the detail Write a main method to create an object of the class and call the above methods.
Write a program by using class with the following specifications:
Class name — Characters
Data Members:
- String str — To store the string
Member Methods:
- void input (String st) — to assign st to str
- void check_print() — to check and print the following:
(i) number of letters
(ii) number of digits
(iii) number of uppercase characters
(iv) number of lowercase characters
(v) number of special characters
Define a class Student with the following specifications:
Class name : Student
Data Members Purpose String name To store the name of the student int eng To store marks in English int hn To store marks in Hindi int mts To store marks in Maths double total To store total marks double avg To store average marks Member Methods Purpose void accept() To input marks in English, Hindi and Maths void compute() To calculate total marks and average of 3 subjects void display() To show all the details viz. name, marks, total and average Write a program to create an object and invoke the above methods.