Computer Applications
Write a program using a method Palin( ), to check whether a string is a Palindrome or not. A Palindrome is a string that reads the same from the left to right and vice versa.
Sample Input: MADAM, ARORA, ABBA, etc.
Java
User Defined Methods
ICSE 2007
84 Likes
Answer
import java.util.Scanner;
public class KboatStringPalindrome
{
public void palin() {
Scanner in = new Scanner(System.in);
System.out.print("Enter the string: ");
String s = in.nextLine();
String str = s.toUpperCase();
int strLen = str.length();
boolean isPalin = true;
for (int i = 0; i < strLen / 2; i++) {
if (str.charAt(i) != str.charAt(strLen - 1 - i)) {
isPalin = false;
break;
}
}
if (isPalin)
System.out.println("It is a palindrome string.");
else
System.out.println("It is not a palindrome string.");
}
}
Variable Description Table
Program Explanation
Output
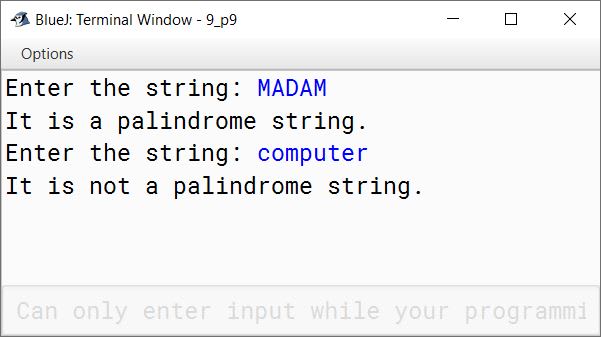
Answered By
30 Likes
Related Questions
Write a program in Java to accept a String from the user. Pass the String to a method Display(String str) which displays the consonants present in the String.
Sample Input: computer
Sample Output:
c
m
p
t
rWrite a program using method name Glcm(int,int) to find the Lowest Common Multiple (LCM) of two numbers by GCD (Greatest Common Divisor) of the numbers. GCD of two integers is calculated by continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process is repeated till the remainder is zero. The divisor then results in the GCD.
LCM = product of two numbers / GCDWrite a program in Java to accept a String from the user. Pass the String to a method Change(String str) which displays the first character of each word after changing the case (lower to upper and vice versa).
Sample Input: Delhi public school
Sample Output:
d
P
SWrite a program in Java to accept a word. Pass it to a method magic(String str). The method checks the string for the presence of consecutive letters. If two letters are consecutive at any position then the method prints "It is a magic string", otherwise it prints "It is not a magic string".
Sample Input: computer
Sample Output: It is not a magic string
Sample Input: DELHI
Sample Output: It is a magic string