Computer Applications
Write a program using method name Glcm(int,int) to find the Lowest Common Multiple (LCM) of two numbers by GCD (Greatest Common Divisor) of the numbers. GCD of two integers is calculated by continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process is repeated till the remainder is zero. The divisor then results in the GCD.
LCM = product of two numbers / GCD
Java
User Defined Methods
57 Likes
Answer
import java.util.Scanner;
public class KboatGlcm
{
public void Glcm(int a, int b) {
int x = a, y = b;
while (y != 0) {
int t = y;
y = x % y;
x = t;
}
int lcm = (a * b) / x;
System.out.println("LCM = " + lcm);
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first number: ");
int x = in.nextInt();
System.out.print("Enter second number: ");
int y = in.nextInt();
KboatGlcm obj = new KboatGlcm();
obj.Glcm(x, y);
}
}
Variable Description Table
Program Explanation
Output
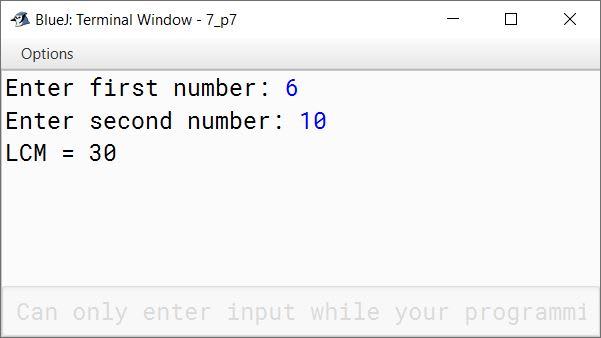
Answered By
21 Likes
Related Questions
Write a program in Java to accept a word. Pass it to a method magic(String str). The method checks the string for the presence of consecutive letters. If two letters are consecutive at any position then the method prints "It is a magic string", otherwise it prints "It is not a magic string".
Sample Input: computer
Sample Output: It is not a magic string
Sample Input: DELHI
Sample Output: It is a magic stringWrite a method fact(int n) to find the factorial of a number n. Include a main class to find the value of S where:
S = n! / (m!(n - m)!)
where, n! = 1 x 2 x 3 x ………. x nWrite a program using a method called area() to compute area of the following:
(a) Area of circle = (22/7) * r * r
(b) Area of square= side * side
(c) Area of rectangle = length * breadth
Display the menu to display the area as per the user's choice.
Write a program using a method Palin( ), to check whether a string is a Palindrome or not. A Palindrome is a string that reads the same from the left to right and vice versa.
Sample Input: MADAM, ARORA, ABBA, etc.