Computer Applications
Write a program in Java to accept a String from the user. Pass the String to a method Display(String str) which displays the consonants present in the String.
Sample Input: computer
Sample Output:
c
m
p
t
r
Java
User Defined Methods
18 Likes
Answer
import java.util.Scanner;
public class KboatConsonants
{
public void display(String str) {
String t = str.toUpperCase();
int len = t.length();
for (int i = 0; i < len; i++) {
char ch = t.charAt(i);
if (ch != 'A' &&
ch != 'E' &&
ch != 'I' &&
ch != 'O' &&
ch != 'U') {
System.out.println(str.charAt(i));
}
}
}
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter string: ");
String s = in.nextLine();
KboatConsonants obj = new KboatConsonants();
obj.display(s);
}
}
Variable Description Table
Program Explanation
Output
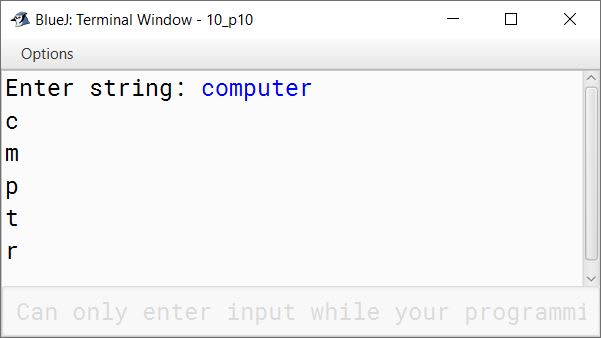
Answered By
9 Likes
Related Questions
Write a program using a method Palin( ), to check whether a string is a Palindrome or not. A Palindrome is a string that reads the same from the left to right and vice versa.
Sample Input: MADAM, ARORA, ABBA, etc.Write a program in Java to accept a String from the user. Pass the String to a method Change(String str) which displays the first character of each word after changing the case (lower to upper and vice versa).
Sample Input: Delhi public school
Sample Output:
d
P
SWrite a program in Java to accept a word. Pass it to a method magic(String str). The method checks the string for the presence of consecutive letters. If two letters are consecutive at any position then the method prints "It is a magic string", otherwise it prints "It is not a magic string".
Sample Input: computer
Sample Output: It is not a magic string
Sample Input: DELHI
Sample Output: It is a magic stringWrite a program in Java to accept the name of an employee and his/her annual income. Pass the name and the annual income to a method Tax(String name, int income) which displays the name of the employee and the income tax as per the given tariff:
Annual Income Income Tax Up to ₹2,50,000 No tax ₹2,50,001 to ₹5,00,000 10% of the income exceeding ₹2,50,000 ₹5,00,001 to ₹10,00,000 ₹30,000 + 20% of the amount exceeding ₹5,00,000 ₹10,00,001 and above ₹50,000 + 30% of the amount exceeding ₹10,00,000