Computer Applications
Write a program using a method called area() to compute area of the following:
(a) Area of circle = (22/7) * r * r
(b) Area of square= side * side
(c) Area of rectangle = length * breadth
Display the menu to display the area as per the user's choice.
Java
User Defined Methods
ICSE 2005
111 Likes
Answer
import java.util.Scanner;
public class KboatMenuArea
{
public void area() {
Scanner in = new Scanner(System.in);
System.out.println("Enter a to calculate area of circle");
System.out.println("Enter b to calculate area of square");
System.out.println("Enter c to calculate area of rectangle");
System.out.print("Enter your choice: ");
char choice = in.next().charAt(0);
switch(choice) {
case 'a':
System.out.print("Enter radius of circle: ");
double r = in.nextDouble();
double ca = (22 / 7.0) * r * r;
System.out.println("Area of circle = " + ca);
break;
case 'b':
System.out.print("Enter side of square: ");
double side = in.nextDouble();
double sa = side * side;
System.out.println("Area of square = " + sa);
break;
case 'c':
System.out.print("Enter length of rectangle: ");
double l = in.nextDouble();
System.out.print("Enter breadth of rectangle: ");
double b = in.nextDouble();
double ra = l * b;
System.out.println("Area of rectangle = " + ra);
break;
default:
System.out.println("Wrong choice!");
}
}
}
Variable Description Table
Program Explanation
Output
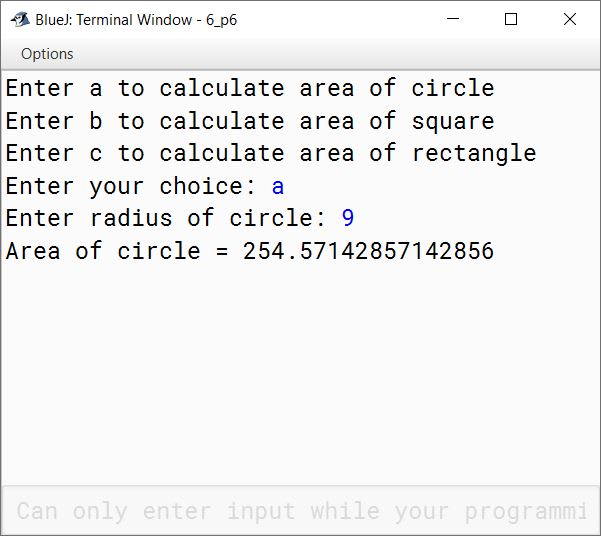
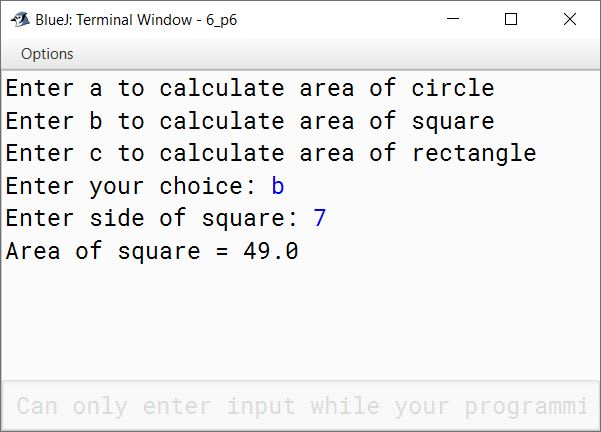
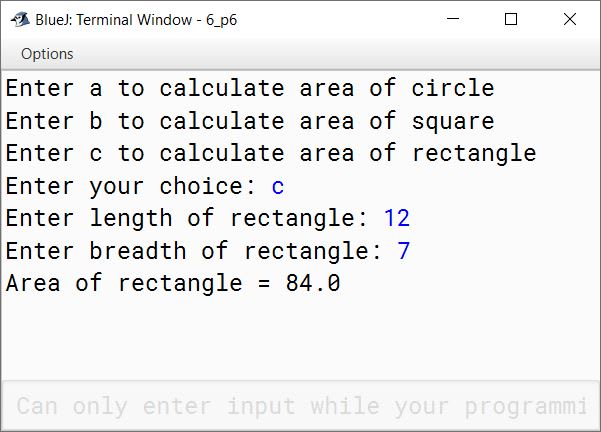
Answered By
34 Likes
Related Questions
Write a program using method name Glcm(int,int) to find the Lowest Common Multiple (LCM) of two numbers by GCD (Greatest Common Divisor) of the numbers. GCD of two integers is calculated by continued division method. Divide the larger number by the smaller, the remainder then divides the previous divisor. The process is repeated till the remainder is zero. The divisor then results in the GCD.
LCM = product of two numbers / GCDWrite a program in Java to accept a word. Pass it to a method magic(String str). The method checks the string for the presence of consecutive letters. If two letters are consecutive at any position then the method prints "It is a magic string", otherwise it prints "It is not a magic string".
Sample Input: computer
Sample Output: It is not a magic string
Sample Input: DELHI
Sample Output: It is a magic stringWrite a program to enter a two digit number and find out its first factor excluding 1 (one). The program then find the second factor (when the number is divided by the first factor) and finally displays both the factors.
Hint: Use a non-return type method as void fact(int n) to accept the number.Sample Input: 21
The first factor of 21 is 3
Sample Output: 3, 7
Sample Input: 30
The first factor of 30 is 2
Sample Output: 2, 15Write a method fact(int n) to find the factorial of a number n. Include a main class to find the value of S where:
S = n! / (m!(n - m)!)
where, n! = 1 x 2 x 3 x ………. x n