Computer Applications
Write a program to input a string and print each word of the string in the reverse order.
Sample Input:
Enter a string: My name is Raman
Sample Output
yM eman si namaR
Java
Java String Handling
9 Likes
Answer
import java.util.Scanner;
public class KboatWordsReverse
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
str += " ";
int len = str.length();
String word = "";
int wLen = 0;
char ch;
for (int i = 0; i < len; i++) {
ch = str.charAt(i);
if (ch != ' ') {
word = word + ch;
}
else {
wLen = word.length();
for(int j = wLen - 1; j >= 0; j--) {
System.out.print(word.charAt(j));
}
System.out.print(' ');
word = "";
}
}
}
}
Output
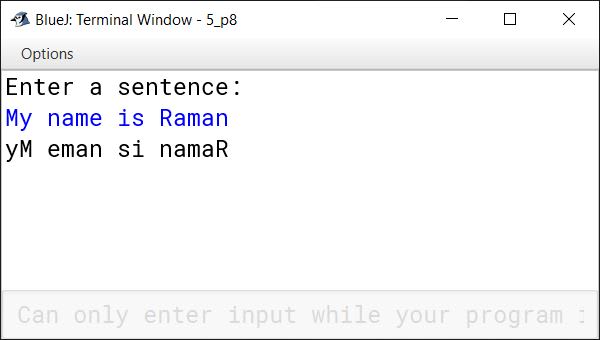
Answered By
3 Likes
Related Questions
Write a program in Java to accept a string and display the number of uppercase, number of lowercase, number of special characters and number of digits present in the string.
Write a program to enter a sentence from the keyboard and count the number of times a particular word occurs in it. Display the frequency of the search word.
Sample Input:
Enter a sentence: The quick brown fox jumps over the lazy dog.
Enter a word to search: theSample Output:
Search word occurs 2 timesWrite a program to input a sentence and arrange words of the string in order of their lengths from shortest to longest.
Write a program to input a sentence. Count and display the frequency of each letter of the sentence in alphabetical order.
Sample Input: COMPUTER APPLICATIONS
Sample Output:Character Frequency Character Frequency A 2 O 2 C 2 P 3 I 1 R 1 L 2 S 1 M 1 T 2 N 1 U 1