Computer Applications
Write a program in Java to accept a string and display the number of uppercase, number of lowercase, number of special characters and number of digits present in the string.
Java
Java String Handling
18 Likes
Answer
import java.util.Scanner;
public class KboatCount
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter a sentence:");
String str = in.nextLine();
int len = str.length();
int uc = 0;
int lc = 0;
int sc = 0;
int dc = 0;
char ch;
for (int i = 0; i < len; i++) {
ch = str.charAt(i);
if (Character.isUpperCase(ch))
uc++;
else if (Character.isLowerCase(ch))
lc++;
else if (Character.isDigit(ch))
dc++;
else if (!Character.isWhitespace(ch))
sc++;
}
System.out.println("UpperCase Count = " + uc);
System.out.println("LowerCase Count = " + lc);
System.out.println("Digit count = " + dc);
System.out.println("Special Character Count = " + sc);
}
}
Output
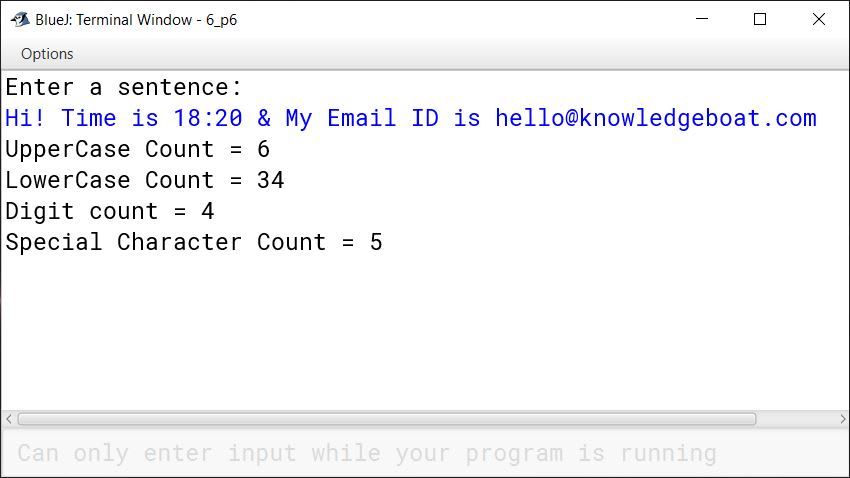
Answered By
6 Likes
Related Questions
Write a program to enter a sentence from the keyboard and count the number of times a particular word occurs in it. Display the frequency of the search word.
Sample Input:
Enter a sentence: The quick brown fox jumps over the lazy dog.
Enter a word to search: theSample Output:
Search word occurs 2 timesWrite a program in Java to enter a String/Sentence and display the longest word and the length of the longest word present in the String.
Sample Input: “TATA FOOTBALL ACADEMY WILL PLAY AGAINST MOHAN BAGAN”
Sample Output: The longest word: FOOTBALL: The length of the word: 8Write a program to input a sentence. Count and display the frequency of each letter of the sentence in alphabetical order.
Sample Input: COMPUTER APPLICATIONS
Sample Output:Character Frequency Character Frequency A 2 O 2 C 2 P 3 I 1 R 1 L 2 S 1 M 1 T 2 N 1 U 1 Write a program to input a string and print each word of the string in the reverse order.
Sample Input:
Enter a string: My name is Raman
Sample Output
yM eman si namaR