Computer Applications
Write a program to enter a sentence from the keyboard and count the number of times a particular word occurs in it. Display the frequency of the search word.
Sample Input:
Enter a sentence: The quick brown fox jumps over the lazy dog.
Enter a word to search: the
Sample Output:
Search word occurs 2 times
Java
Java String Handling
11 Likes
Answer
import java.util.Scanner;
public class KboatWordFrequency
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a sentence: ");
String str = in.nextLine();
System.out.print("Enter a word to search: ");
String ipWord = in.nextLine();
str += " ";
String word = "";
int count = 0;
int len = str.length();
for (int i = 0; i < len; i++) {
if (str.charAt(i) == ' ') {
if (word.equalsIgnoreCase(ipWord))
count++ ;
word = "";
}
else {
word += str.charAt(i);
}
}
if (count > 0) {
System.out.println("Search word occurs " + count + " times.");
}
else {
System.out.println("Search word is not present in sentence.");
}
}
}
Output
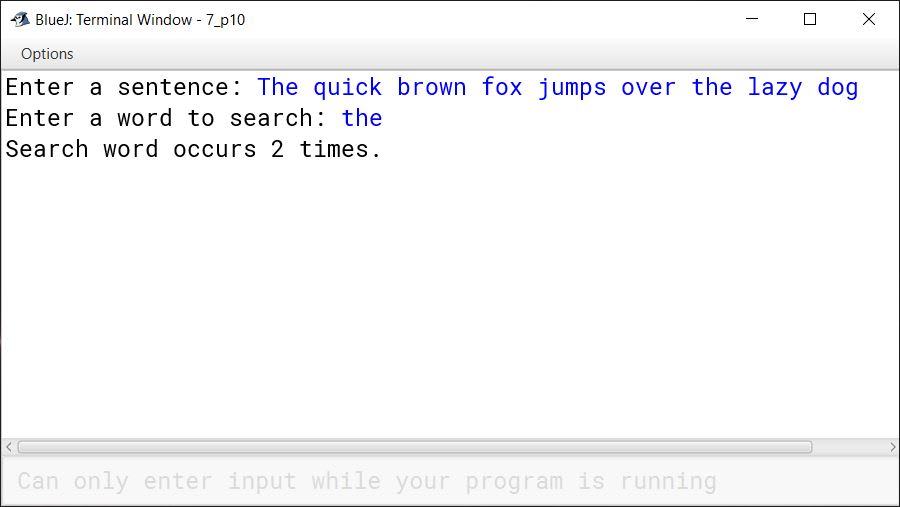
Answered By
6 Likes
Related Questions
Write a program to input a string and print each word of the string in the reverse order.
Sample Input:
Enter a string: My name is Raman
Sample Output
yM eman si namaRWrite a program to accept a string. Convert the string into upper case letters. Count and output the number of double letter sequences that exist in the string.
Sample Input: "SHE WAS FEEDING THE LITTLE RABBIT WITH AN APPLE"
Sample Output: 4Write a program in Java to accept a string and display the number of uppercase, number of lowercase, number of special characters and number of digits present in the string.
Write a program in Java to enter a String/Sentence and display the longest word and the length of the longest word present in the String.
Sample Input: “TATA FOOTBALL ACADEMY WILL PLAY AGAINST MOHAN BAGAN”
Sample Output: The longest word: FOOTBALL: The length of the word: 8