Computer Applications
Write a program to input a number and find whether the number is an emirp number or not. A number is said to be emirp if the original number and the reversed number both are prime numbers.
For example, 17 is an emirp number as 17 and its reverse 71 are both prime numbers.
Java
Java Iterative Stmts
19 Likes
Answer
import java.util.Scanner;
public class KboatEmirp
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter Number: ");
int num = in.nextInt();
int c = 0;
for (int i = 1; i <= num; i++) {
if (num % i == 0) {
c++;
}
}
if (c == 2) {
int t = num;
int revNum = 0;
while(t != 0) {
int digit = t % 10;
t /= 10;
revNum = revNum * 10 + digit;
}
int c2 = 0;
for (int i = 1; i <= revNum; i++) {
if (revNum % i == 0) {
c2++;
}
}
if (c2 == 2)
System.out.println("Emirp Number");
else
System.out.println("Not Emirp Number");
}
else
System.out.println("Not Emirp Number");
}
}
Output
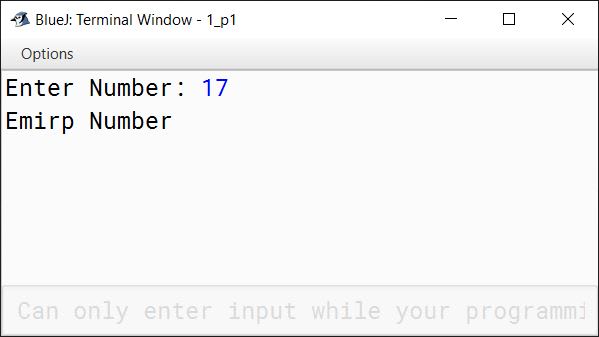
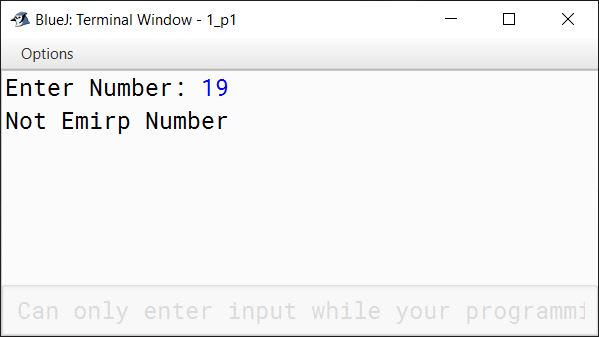
Answered By
3 Likes