Computer Applications
Define a class to accept a four digit number and check whether it is a Framul Number or not. A number is called a Framul Number if the product of all its digits equals fives times the sum of its first and last digit.
Example 1:
Input: 1325
Product of all digits = 1 x 3 x 2 x 5 = 30
Five times sum of first & last digit = 5 x (5 + 1) = 5 x 6 = 30
Output: Framul Number
Example 2:
Input: 2981
Product of all digits = 2 x 9 x 8 x 1 = 144
Five times sum of first & last digit = 5 x (1 + 2) = 5 x 3 = 15
Output: Not a Framul Number
If the entered number is not of four digits, the message "Please enter a four digit number" should be shown.
import java.util.Scanner;
public class KboatFramulNumber
{
public static void main(String args[]) {
Scanner sc = _____(1)_____
System.out.print("Enter a four-digit number: ");
int num = _____(2)_____
_______(3)_______ {
System.out.println("Please enter a four-digit number");
return;
}
int d = 0;
int f = num % 10;
int p = 1;
_______(4)_______ {
_____(5)_____
_____(6)_____
_____(7)_____
}
int s = _____(8)_____
if (p == s) {
_______(9)_______
} else {
_______(10)_______
}
}
}
Java Iterative Stmts
2 Likes
Answer
new Scanner(System.in);
sc.nextInt();
if (num < 1000 || num > 9999)
while (num > 0)
d = num % 10;
p = p * d;
num = num / 10;
5 * (f + d);
System.out.println("Framul Number");
System.out.println("Not a Framul Number");
Explanation
import java.util.Scanner;
public class KboatFramulNumber
{
public static void main(String args[]) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter a four-digit number: ");
int num = sc.nextInt();
if (num < 1000 || num > 9999) {
System.out.println("Please enter a four-digit number");
return;
}
int d = 0;
int f = num % 10;
int p = 1;
while (num > 0) {
d = num % 10;
p = p * d;
num = num / 10;
}
int s = 5 * (f + d);
if (p == s) {
System.out.println("Framul Number");
} else {
System.out.println("Not a Framul Number");
}
}
}
Variable Description Table
Variable Name | Type | Purpose |
---|---|---|
sc | Scanner | An instance of Scanner used to read input from the user. |
num | int | The four-digit number entered by the user to check if it is a Framul Number. |
d | int | The current digit extracted from the number, used in both product calculation and to identify the last digit. |
f | int | The last digit of the original number, used in the five times sum calculation. |
p | int | The product of all digits of the number, calculated iteratively. |
s | int | Five times the sum of the first and last digit of the original number, used to determine if the number is a Framul Number. |
Program Explanation
Let's go through the program step-by-step to understand the logic and functionality:
1. Input Handling:
The program begins by prompting the user to enter a four-digit number. It uses Scanner
to read an integer input from the user.
2. Validation:
if (num < 1000 || num > 9999) {
System.out.println("Please enter a four-digit number");
return;
}
Here, the method checks if the entered number is indeed a four-digit number. If num
is less than 1000 or greater than 9999, it prints a message asking for a four-digit number and exits the method.
3. Initialization:
int d = 0;
int f = num % 10;
int p = 1;
d
is initialized to store each digit during the extraction process.f
is initialized to hold the last digit of the number (num % 10
).p
is initialized to 1 for calculating the product of digits.
4. Digit Extraction and Product Calculation:
while (num > 0) {
d = num % 10;
p = p * d;
num = num / 10;
}
- A
while
loop iteratively extracts each digit of the number. d = num % 10
extracts the current last digit.p = p * d
updates the running product with the current digit.num = num / 10
removes the last digit from the number.
5. Calculate Sum and Comparison:
int s = 5 * (f + d);
s
is calculated as five times the sum of the first (d
from the last iteration) and last (f
) digit.
6. Determine Framul Number:
if (p == s) {
System.out.println("Framul Number");
} else {
System.out.println("Not a Framul Number");
}
- The program compares the product of the digits (
p
) withs
. - If they are equal, it outputs "Framul Number"; otherwise, "Not a Framul Number".
Output
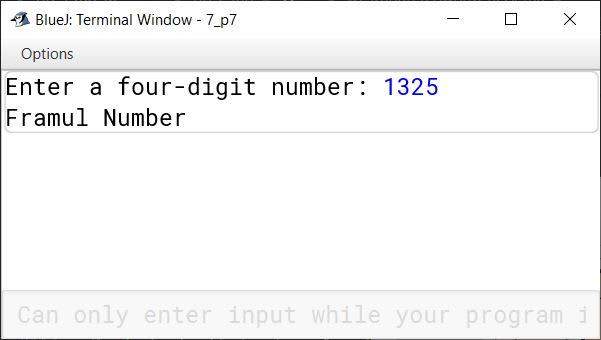
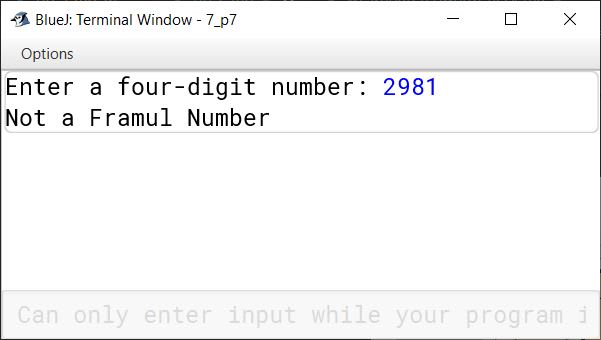
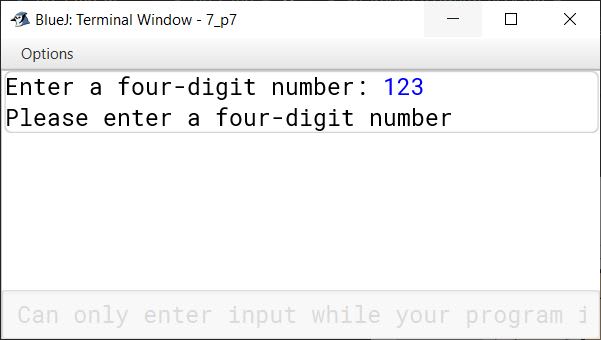
Answered By
2 Likes
Related Questions
How many times will the following loop execute? Write the output of the code:
int a = 5; while (a > 0) { System.out.println(a-- + 2); if (a % 3 == 0) break; }
Rewrite the following do while program segment using for:
x = 10; y = 20; do { x++; y++; } while (x<=20); System.out.println(x * y );
To execute a loop 5 times, which of the following is correct?
How many times will the following loop execute? Write the output of the code:
int x=10; while (true){ System.out.println(x++ * 2); if(x%3==0) break; }