Computer Applications
Write a program to input a number and check whether it is 'Magic Number' or not. Display the message accordingly.
A number is said to be a magic number if the eventual sum of digits of the number is one.
Sample Input : 55
Then, 5 + 5 = 10, 1 + 0 = 1
Sample Output: Hence, 55 is a Magic Number.
Similarly, 289 is a Magic Number.
Java
Java Nested for Loops
140 Likes
Answer
import java.util.Scanner;
public class KboatMagicNum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number to check: ");
int num = in.nextInt();
int n = num;
while (n > 9) {
int sum = 0;
while (n != 0) {
int d = n % 10;
n /= 10;
sum += d;
}
n = sum;
}
if (n == 1)
System.out.println(num + " is Magic Number");
else
System.out.println(num + " is not Magic Number");
}
}
Variable Description Table
Program Explanation
Output
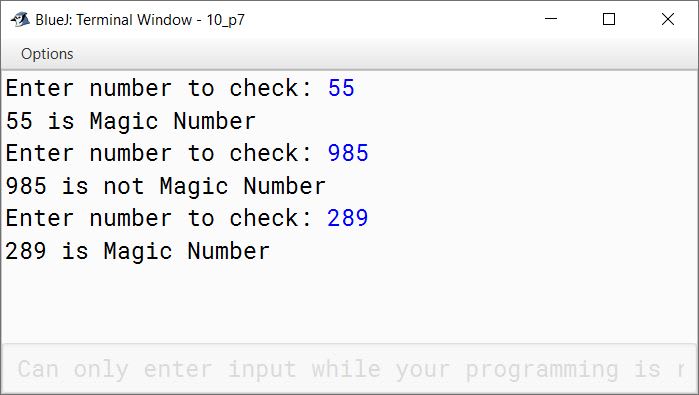
Answered By
45 Likes
Related Questions
In an entrance examination, students have been appeared in English, Maths and Science papers. Write a program to calculate and display average marks obtained by all the students. Take number of students appeared and marks obtained in all three subjects by every student along with the name as inputs.
Display the name, marks obtained in three subjects and the average of all the students.Write a program in Java to enter a number containing three digits or more. Arrange the digits of the entered number in ascending order and display the result.
Sample Input: Enter a number 4972
Sample Output: 2, 4, 7, 9A number is said to be Multiple Harshad number, when divided by the sum of its digits, produces another 'Harshad Number'. Write a program to input a number and check whether it is a Multiple Harshad Number or not.
(When a number is divisible by the sum of its digit, it is called 'Harshad Number').
Sample Input: 6804
Hint: 6804 ⇒ 6+8+0+4 = 18 ⇒ 6804/18 = 378
378 ⇒ 3+7+8= 18 ⇒ 378/18 = 21
21 ⇒ 2+1 = 3 ⇒ 21/3 = 7
Sample Output: Multiple Harshad NumberWrite a program in Java to display the following pattern:
1
3 1
5 3 1
7 5 3 1
9 7 5 3 1