Computer Applications
A number is said to be Multiple Harshad number, when divided by the sum of its digits, produces another 'Harshad Number'. Write a program to input a number and check whether it is a Multiple Harshad Number or not.
(When a number is divisible by the sum of its digit, it is called 'Harshad Number').
Sample Input: 6804
Hint: 6804 ⇒ 6+8+0+4 = 18 ⇒ 6804/18 = 378
378 ⇒ 3+7+8= 18 ⇒ 378/18 = 21
21 ⇒ 2+1 = 3 ⇒ 21/3 = 7
Sample Output: Multiple Harshad Number
Java
Java Nested for Loops
42 Likes
Answer
import java.util.Scanner;
public class KboatMultipleHarshad
{
public void checkMultipleHarshad() {
Scanner in = new Scanner(System.in);
System.out.print("Enter number to check: ");
int num = in.nextInt();
int dividend = num;
int divisor;
int count = 0;
while (dividend > 1) {
divisor=0;
int t = dividend;
while (t > 0) {
int d = t % 10;
divisor += d;
t /= 10;
}
if (dividend % divisor == 0 && divisor != 1) {
dividend = dividend / divisor;
count++;
}
else {
break;
}
}
if (dividend == 1 && count > 1)
System.out.println(num + " is Multiple Harshad Number");
else
System.out.println(num + " is not Multiple Harshad Number");
}
}
Variable Description Table
Program Explanation
Output
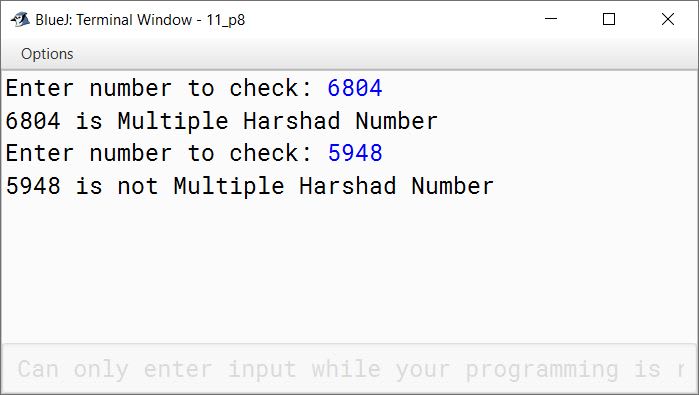
Answered By
13 Likes
Related Questions
Write a program in Java to display the following pattern:
1 2 3 4 5
6 7 8 9
10 11 12
13 14
15Write a program to input a number and check whether it is 'Magic Number' or not. Display the message accordingly.
A number is said to be a magic number if the eventual sum of digits of the number is one.
Sample Input : 55
Then, 5 + 5 = 10, 1 + 0 = 1
Sample Output: Hence, 55 is a Magic Number.
Similarly, 289 is a Magic Number.Write a program in Java to enter a number containing three digits or more. Arrange the digits of the entered number in ascending order and display the result.
Sample Input: Enter a number 4972
Sample Output: 2, 4, 7, 9Write a program in Java to display the following pattern:
1
3 1
5 3 1
7 5 3 1
9 7 5 3 1