Computer Applications
In an entrance examination, students have been appeared in English, Maths and Science papers. Write a program to calculate and display average marks obtained by all the students. Take number of students appeared and marks obtained in all three subjects by every student along with the name as inputs.
Display the name, marks obtained in three subjects and the average of all the students.
Java
Java Nested for Loops
50 Likes
Answer
import java.util.Scanner;
public class KboatStudentMarks
{
public void studentMarks() {
Scanner in = new Scanner(System.in);
System.out.print("Enter number of students: ");
int studentCount = in.nextInt();
String names[] = new String[studentCount];
int engMarks[] = new int[studentCount];
int sciMarks[] = new int[studentCount];
int mathsMarks[] = new int[studentCount];
double avgMarks[] = new double[studentCount];
double totalMarks = 0.0;
for (int i = 0; i < studentCount; i++) {
System.out.println("Enter details of student " + (i + 1));
System.out.print("Name: ");
in.nextLine();
names[i] = in.nextLine();
System.out.print("Marks in English: ");
engMarks[i] = in.nextInt();
System.out.print("Marks in Science: ");
sciMarks[i] = in.nextInt();
System.out.print("Marks in Maths: ");
mathsMarks[i] = in.nextInt();
avgMarks[i] = (engMarks[i] + sciMarks[i] + mathsMarks[i]) / 3.0;
totalMarks += avgMarks[i];
}
System.out.println();
for (int i = 0; i < studentCount; i++) {
System.out.println("Details of student " + (i + 1));
System.out.println("Name: " + names[i]);
System.out.println("English: " + engMarks[i]);
System.out.println("Science: " + sciMarks[i]);
System.out.println("Maths: " + mathsMarks[i]);
System.out.println("Average: " + avgMarks[i]);
}
double classAvg = totalMarks / studentCount;
System.out.println("\nAverage of all students is " + classAvg);
}
}
Variable Description Table
Program Explanation
Output
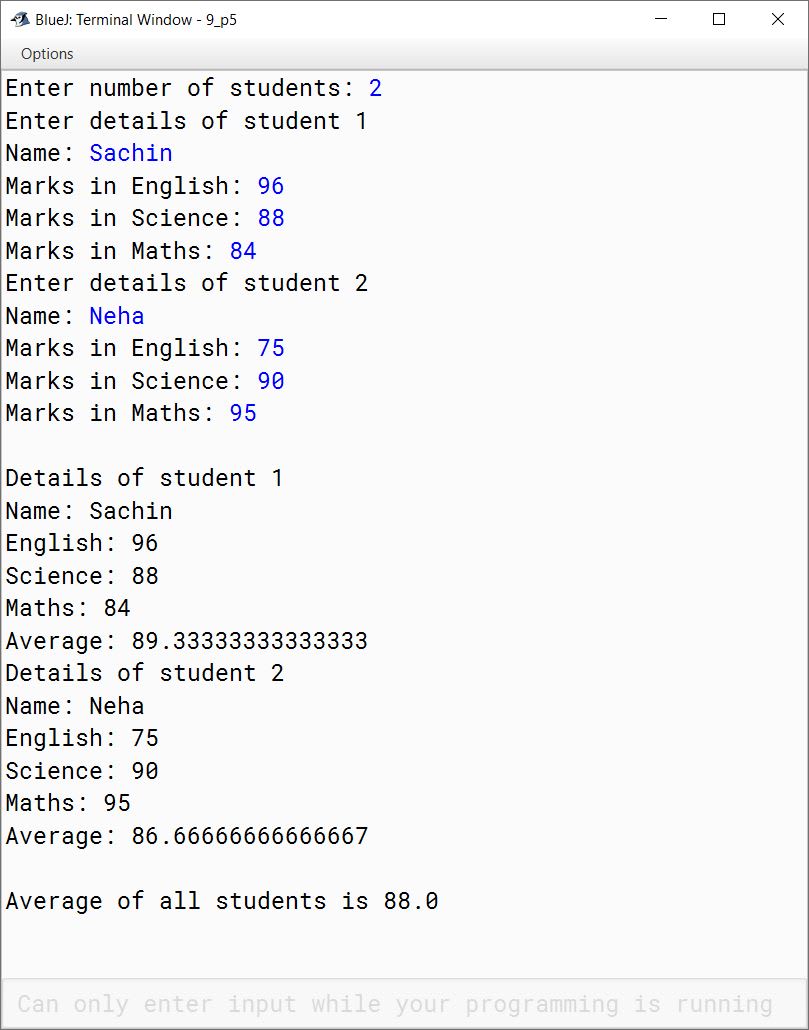
Answered By
17 Likes
Related Questions
Write a program to display all the numbers between 100 and 200 which don't contain zeros at any position.
For example: 111, 112, 113, ……. , 199Write a program to display all prime palindrome numbers between 10 and 1000.
[Hint: A number which is prime as well a palindrome is said to be 'Prime Palindrome' number.]
For example: 11, 101, 131, 151,Write a program in Java to enter a number containing three digits or more. Arrange the digits of the entered number in ascending order and display the result.
Sample Input: Enter a number 4972
Sample Output: 2, 4, 7, 9Write a program to input a number and check whether it is 'Magic Number' or not. Display the message accordingly.
A number is said to be a magic number if the eventual sum of digits of the number is one.
Sample Input : 55
Then, 5 + 5 = 10, 1 + 0 = 1
Sample Output: Hence, 55 is a Magic Number.
Similarly, 289 is a Magic Number.