Computer Applications
Write a program to input a letter. Find its ASCII code. Reverse the ASCII code and display the equivalent character.
Sample Input: Y
Sample Output: ASCII Code = 89
Reverse the code = 98
Equivalent character: b
Java
Java Library Classes
83 Likes
Answer
import java.util.Scanner;
public class KboatASCIIReverse
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter a letter: ");
char l = in.next().charAt(0);
int a = (int)l;
System.out.println("ASCII Code = " + a);
int r = 0;
while (a > 0) {
int digit = a % 10;
r = r * 10 + digit;
a /= 10;
}
System.out.println("Reversed Code = " + r);
System.out.println("Equivalent character = " + (char)r);
}
}
Variable Description Table
Program Explanation
Output
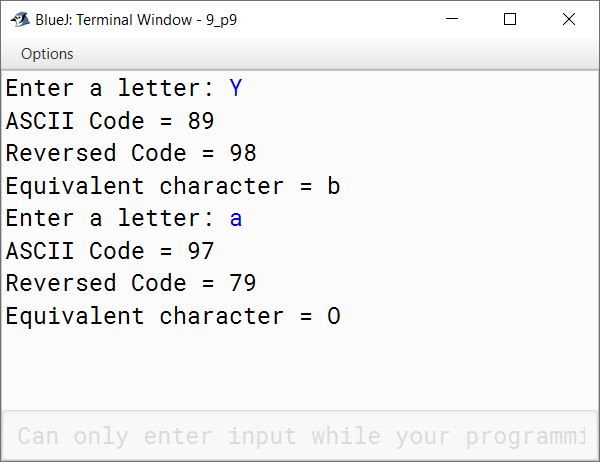
Answered By
30 Likes
Related Questions
Using switch case statement, write a menu driven program to perform the following tasks:
(a) To generate and print the letters from A to Z along with their Unicode.
Letters Unicode
A 65
B 66
….. ……..
….. ……..
Z 90(b) To generate and print the letters from z to a along with their Unicode.
Letters Unicode
z 122
y 121
….. ……..
….. ……..
a 97Write a menu driven program to display
(i) first five upper case letters
(ii) last five lower case letters as per the user's choice.
Enter '1' to display upper case letters and enter '2' to display lower case letters.Write a program to input a set of any 10 integer numbers. Find the sum and product of the numbers. Join the sum and product to form a single number. Display the concatenated number.
[Hint: let sum=245 and product = 1346 then the number after joining sum and product will be 2451346]Write a menu driven program to generate the upper case letters from Z to A and lower case letters from 'a' to 'z' as per the user's choice.
Enter '1' to display upper case letters from Z to A and Enter '2' to display lower case letters from a to z.