Computer Applications
Using switch case statement, write a menu driven program to perform the following tasks:
(a) To generate and print the letters from A to Z along with their Unicode.
Letters Unicode
A 65
B 66
….. ……..
….. ……..
Z 90
(b) To generate and print the letters from z to a along with their Unicode.
Letters Unicode
z 122
y 121
….. ……..
….. ……..
a 97
Java
Java Library Classes
16 Likes
Answer
import java.util.Scanner;
public class KboatLettersNUnicode
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter 1 for A to Z with unicode");
System.out.println("Enter 2 for z to a with unicode");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
System.out.println("Letters" + "\t" + "Unicode");
for(int i = 65; i <= 90; i++)
System.out.println((char)i + "\t" + i);
break;
case 2:
System.out.println("Letters" + "\t" + "Unicode");
for (int i = 122; i >= 97; i--)
System.out.println((char)i + "\t" + i);
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Variable Description Table
Program Explanation
Output
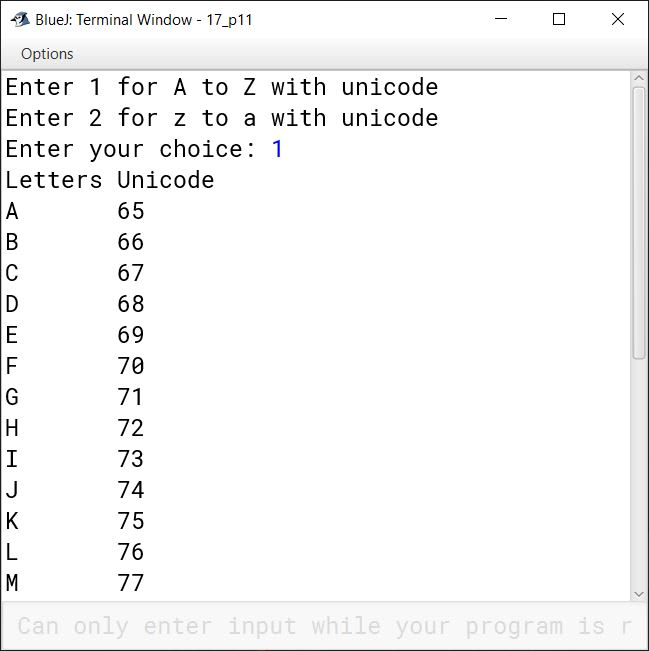
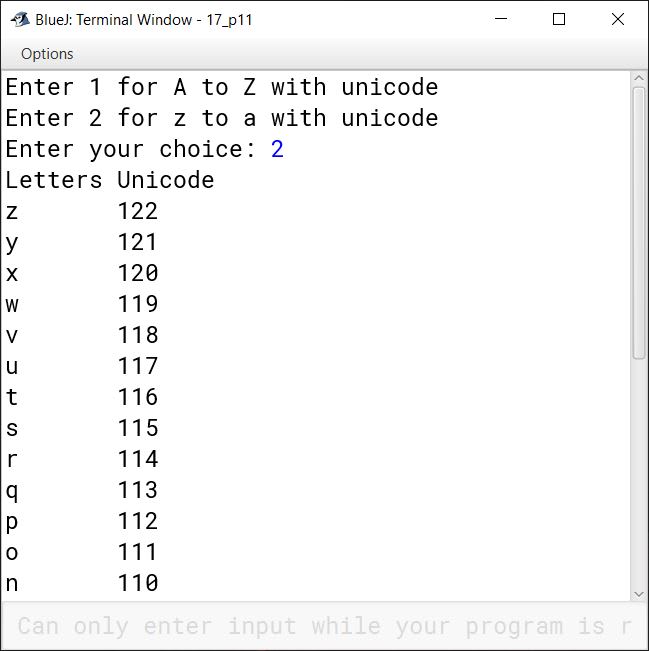
Answered By
7 Likes
Related Questions
Write a menu driven program to display
(i) first five upper case letters
(ii) last five lower case letters as per the user's choice.
Enter '1' to display upper case letters and enter '2' to display lower case letters.Write a program to input a letter. Find its ASCII code. Reverse the ASCII code and display the equivalent character.
Sample Input: Y
Sample Output: ASCII Code = 89
Reverse the code = 98
Equivalent character: bWrite a program in Java to display the following pattern:
A
ab
ABC
abcd
ABCDEWrite a program in Java to display the following pattern:
ZYXWU
ZYXW
ZYX
ZY
Z