Computer Applications
Write a menu driven program to generate the upper case letters from Z to A and lower case letters from 'a' to 'z' as per the user's choice.
Enter '1' to display upper case letters from Z to A and Enter '2' to display lower case letters from a to z.
Java
Java Library Classes
56 Likes
Answer
import java.util.Scanner;
public class KboatLetters
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Enter '1' to display upper case letters from Z to A");
System.out.println("Enter '2' to display lower case letters from a to z");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
int count = 0;
switch (ch) {
case 1:
for (int i = 90; i > 64; i--) {
char c = (char)i;
System.out.print(c);
System.out.print(" ");
count++;
//Print 10 characters per line
if (count == 10) {
System.out.println();
count = 0;
}
}
break;
case 2:
for (int i = 97; i < 123; i++) {
char c = (char)i;
System.out.print(c);
System.out.print(" ");
count++;
//Print 10 characters per line
if (count == 10) {
System.out.println();
count = 0;
}
}
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Variable Description Table
Program Explanation
Output
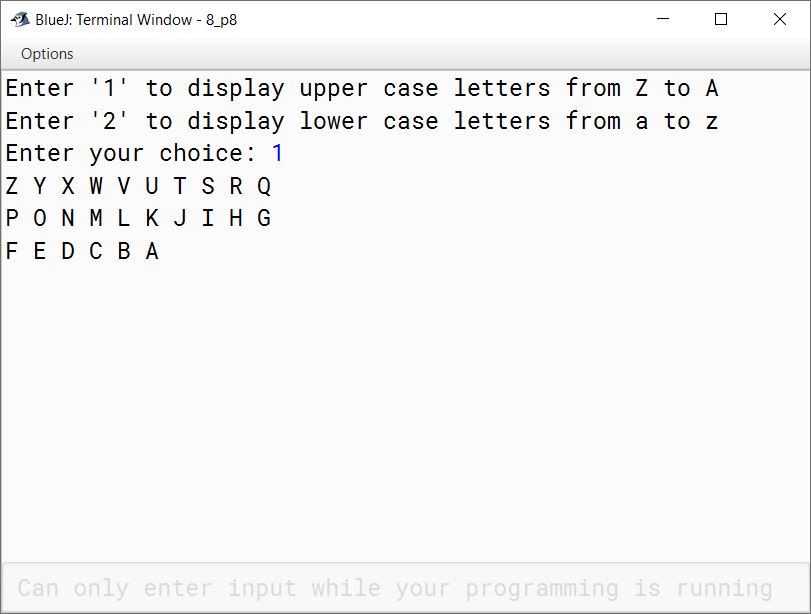
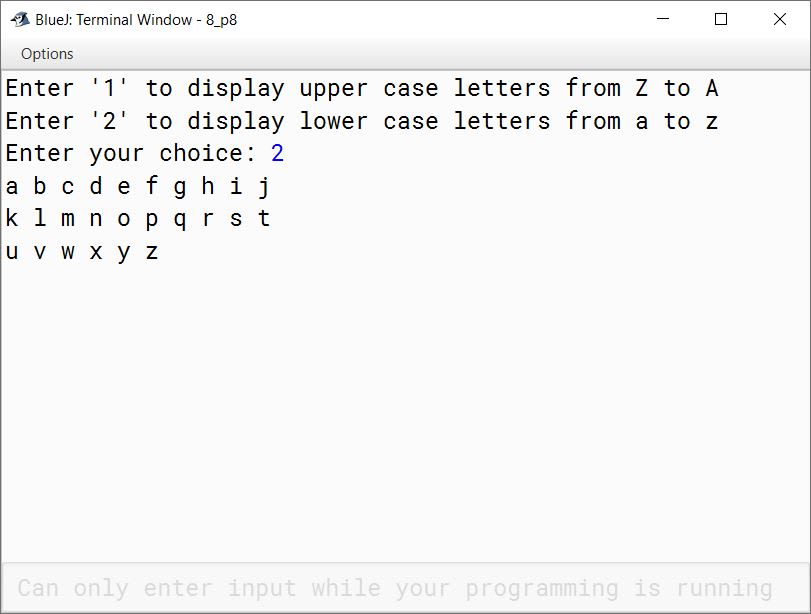
Answered By
20 Likes
Related Questions
Write a program to input two characters from the keyboard. Find the difference (d) between their ASCII codes. Display the following messages:
If d = 0 : both the characters are same.
If d \< 0 : first character is smaller.
If d > 0 : second character is smaller.
Sample Input :
D
P
Sample Output :
d = (68 - 80) = -12
First character is smallerWrite a menu driven program to display
(i) first five upper case letters
(ii) last five lower case letters as per the user's choice.
Enter '1' to display upper case letters and enter '2' to display lower case letters.Write a program to input a set of any 10 integer numbers. Find the sum and product of the numbers. Join the sum and product to form a single number. Display the concatenated number.
[Hint: let sum=245 and product = 1346 then the number after joining sum and product will be 2451346]Write a program to input a letter. Find its ASCII code. Reverse the ASCII code and display the equivalent character.
Sample Input: Y
Sample Output: ASCII Code = 89
Reverse the code = 98
Equivalent character: b