Computer Applications
Write a program to input two characters from the keyboard. Find the difference (d) between their ASCII codes. Display the following messages:
If d = 0 : both the characters are same.
If d \< 0 : first character is smaller.
If d > 0 : second character is smaller.
Sample Input :
D
P
Sample Output :
d = (68 - 80) = -12
First character is smaller
Java
Java Library Classes
51 Likes
Answer
import java.util.Scanner;
public class KboatASCIIDiff
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter first character: ");
char ch1 = in.next().charAt(0);
System.out.print("Enter second character: ");
char ch2 = in.next().charAt(0);
int d = (int)ch1 - (int)ch2;
if (d > 0)
System.out.println("Second character is smaller");
else if (d < 0)
System.out.println("First character is smaller");
else
System.out.println("Both the characters are same");
}
}
Variable Description Table
Program Explanation
Output
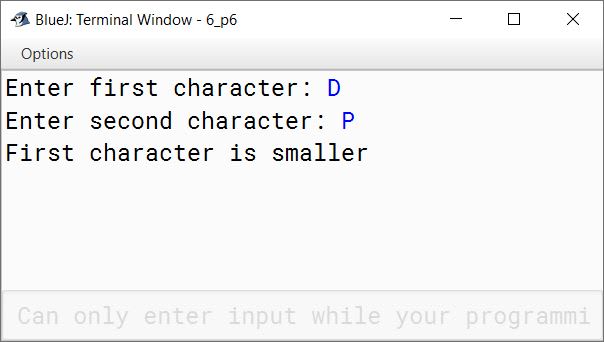
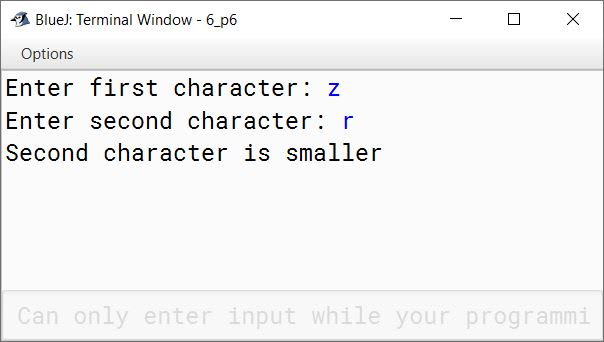
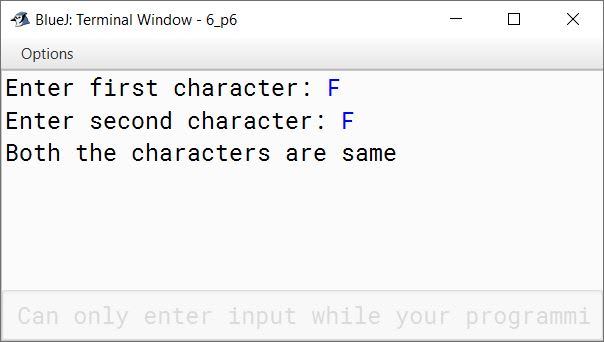
Answered By
16 Likes
Related Questions
Write a menu driven program to generate the upper case letters from Z to A and lower case letters from 'a' to 'z' as per the user's choice.
Enter '1' to display upper case letters from Z to A and Enter '2' to display lower case letters from a to z.Write a program to input a set of 20 letters. Convert each letter into upper case. Find and display the number of vowels and number of consonants present in the set of given letters.
Write a program in Java to accept an integer number N such that 0<N<27. Display the corresponding letter of the alphabet (i.e. the letter at position N).
[Hint: If N =1 then display A]Write a program to input a set of any 10 integer numbers. Find the sum and product of the numbers. Join the sum and product to form a single number. Display the concatenated number.
[Hint: let sum=245 and product = 1346 then the number after joining sum and product will be 2451346]