Computer Science
Write a program to create a double dimensional array of size n x m. Input the numbers in first (n-1) x (m-1) cells. Find and place the sum of each row and each column in corresponding cells of last column and last row respectively. Finally, display the array elements along with the sum of rows and columns.
Sample Input
10 | 15 | 16 | 18 | |
15 | 14 | 12 | 11 | |
11 | 12 | 16 | 17 | |
12 | 10 | 14 | 16 | |
Sample Output
10 | 15 | 16 | 18 | 59 |
15 | 14 | 12 | 11 | 52 |
11 | 12 | 16 | 17 | 56 |
12 | 10 | 14 | 16 | 52 |
48 | 51 | 58 | 62 |
Java
Java Arrays
9 Likes
Answer
import java.util.Scanner;
public class KboatDDASum
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number of rows (n): ");
int n = in.nextInt();
System.out.print("Enter number of columns (m): ");
int m = in.nextInt();
int arr[][] = new int[n][m];
System.out.println("Enter array elements");
for (int i = 0; i < n - 1; i++) {
System.out.println("Enter Row "+ (i+1) + " :");
for (int j = 0; j < m - 1; j++) {
arr[i][j] = in.nextInt();
}
}
System.out.println("Input Array:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
//Row-wise & Column-wise Sum
for (int i = 0; i < n - 1; i++) {
int rSum = 0, cSum = 0;
for (int j = 0; j < m - 1; j++) {
rSum += arr[i][j];
cSum += arr[j][i];
}
arr[i][m - 1] = rSum;
arr[n - 1][i] = cSum;
}
System.out.println("Array with Sum:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
}
Output
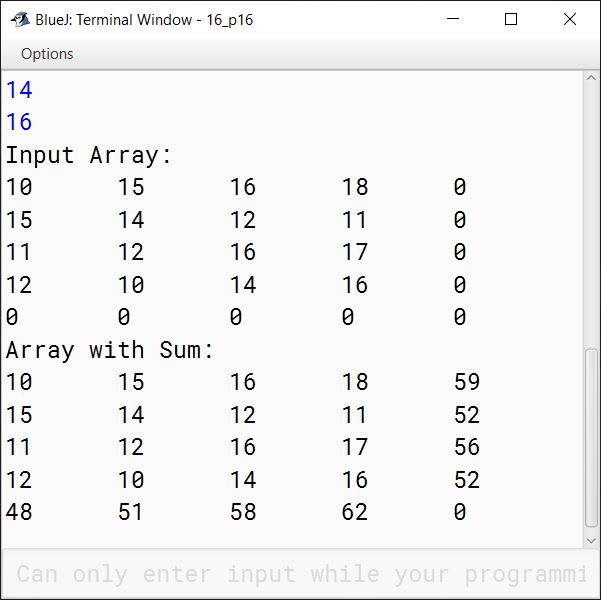
Answered By
4 Likes
Related Questions
Write a program in Java to enter natural numbers in a double dimensional array m x n (where m is the number of rows and n is the number of columns). Display the new matrix in such a way that the new matrix is the mirror image of the original matrix.
8 15 9 18 9 10 7 6 10 8 11 13 12 16 17 19 Sample Input 18 9 15 8 6 7 10 9 13 11 8 10 19 17 16 12 Sample Output Write a Program in Java to fill a 2D array with the first 'mxn' prime numbers, where 'm' is the number of rows and 'n' is the number of columns.
For example:
If rows = 4 and columns = 5, then the result should be:2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 Write a program in Java to create a 4 x 4 matrix. Now, swap the elements of 0th row with 3rd row correspondingly. Display the result after swapping.
Sample Input
55 33 26 14 81 86 31 10 58 64 17 12 22 14 23 25 Sample Output
22 14 23 25 81 86 31 10 58 64 17 12 55 33 26 14 Write a program in Java to store the elements in two different double dimensional arrays (in matrix form) A and B each of order 4 x 4. Find the product of both the matrices and store the result in matrix C. Display the elements of matrix C.
Note:
Two matrixes can be multiplied only if the number of columns of the first matrix must be equal to the number of rows of the second matrix.Sample Input: Matrix A
3 2 1 2 6 4 5 0 7 -1 0 2 4 3 1 1 Sample Input: Matrix B
-2 -4 -1 0 3 6 -5 2 5 3 4 6 0 -2 2 5 Sample Output: Matrix C
5 -1 -5 20 25 15 -6 38 -17 -38 2 -8 6 3 -13 17