Computer Science
Write a Program in Java to fill a 2D array with the first 'mxn' prime numbers, where 'm' is the number of rows and 'n' is the number of columns.
For example:
If rows = 4 and columns = 5, then the result should be:
2 | 3 | 5 | 7 | 11 |
13 | 17 | 19 | 23 | 29 |
31 | 37 | 41 | 43 | 47 |
53 | 59 | 61 | 67 | 71 |
Java
Java Arrays
19 Likes
Answer
import java.util.Scanner;
public class KboatSDAPrime
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number of rows (m): ");
int m = in.nextInt();
System.out.print("Enter number of columns (n): ");
int n = in.nextInt();
int arr[][] = new int[m][n];
int r = 0, c = 0;
int total = m * n;
int count = 0;
for (int i = 2; count < total; i++) {
int div = 0;
for (int j = 1; j <= i; j++) {
if (i % j == 0) {
div++;
}
}
if (div == 2) {
arr[r][c++] = i;
count++;
if (c == n) {
r++;
c = 0;
}
}
}
System.out.println("Prime Numbers Array:");
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
}
Output
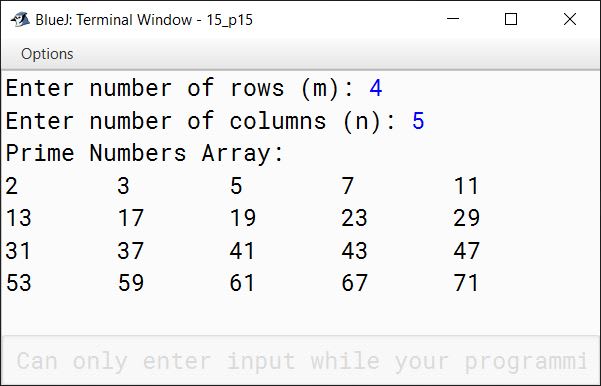
Answered By
5 Likes
Related Questions
Write a program in Java to enter natural numbers in a double dimensional array mxn (where m is the number of rows and n is the number of columns). Shift the elements of 4th column into the 1st column, the elements of 1st column into the 2nd column and so on. Display the new matrix.
11 16 7 4 8 10 9 18 9 8 12 15 14 15 13 6 Sample Input 4 11 16 7 18 8 10 9 15 9 8 12 6 14 15 13 Sample Output Write a program in Java to enter natural numbers in a double dimensional array m x n (where m is the number of rows and n is the number of columns). Display the new matrix in such a way that the new matrix is the mirror image of the original matrix.
8 15 9 18 9 10 7 6 10 8 11 13 12 16 17 19 Sample Input 18 9 15 8 6 7 10 9 13 11 8 10 19 17 16 12 Sample Output Write a program in Java to create a 4 x 4 matrix. Now, swap the elements of 0th row with 3rd row correspondingly. Display the result after swapping.
Sample Input
55 33 26 14 81 86 31 10 58 64 17 12 22 14 23 25 Sample Output
22 14 23 25 81 86 31 10 58 64 17 12 55 33 26 14 Write a program to create a double dimensional array of size n x m. Input the numbers in first (n-1) x (m-1) cells. Find and place the sum of each row and each column in corresponding cells of last column and last row respectively. Finally, display the array elements along with the sum of rows and columns.
Sample Input
10 15 16 18 15 14 12 11 11 12 16 17 12 10 14 16 Sample Output
10 15 16 18 59 15 14 12 11 52 11 12 16 17 56 12 10 14 16 52 48 51 58 62