Computer Science
Write a program in Java to create a 4 x 4 matrix. Now, swap the elements of 0th row with 3rd row correspondingly. Display the result after swapping.
Sample Input
55 | 33 | 26 | 14 |
81 | 86 | 31 | 10 |
58 | 64 | 17 | 12 |
22 | 14 | 23 | 25 |
Sample Output
22 | 14 | 23 | 25 |
81 | 86 | 31 | 10 |
58 | 64 | 17 | 12 |
55 | 33 | 26 | 14 |
Java
Java Arrays
17 Likes
Answer
import java.util.Scanner;
public class KboatDDARowSwap
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
int arr[][] = new int[4][4];
System.out.println("Enter elements of 4x4 array ");
for (int i = 0; i < 4; i++) {
System.out.println("Enter Row "+ (i+1) + " :");
for (int j = 0; j < 4; j++) {
arr[i][j] = in.nextInt();
}
}
System.out.println("Input Array:");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
//Swap 0th and 3rd rows
for (int j = 0; j < 4; j++) {
int t = arr[0][j];
arr[0][j] = arr[3][j];
arr[3][j] = t;
}
System.out.println("Swapped Array:");
for (int i = 0; i < 4; i++) {
for (int j = 0; j < 4; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
}
}
Output
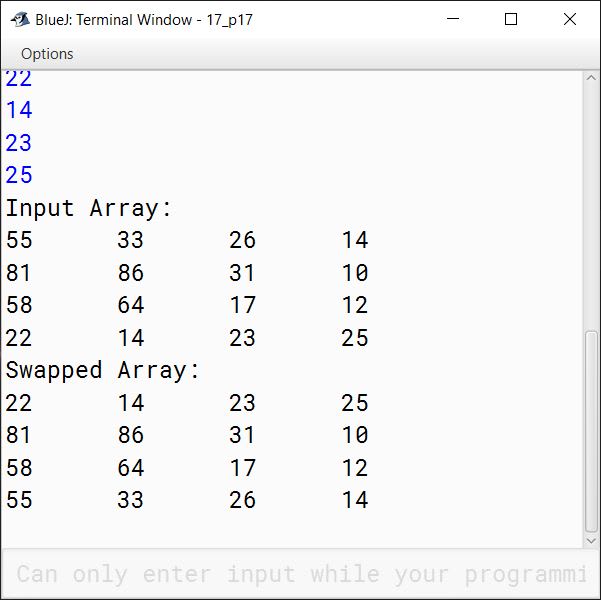
Answered By
5 Likes
Related Questions
Write a program to create a double dimensional array of size n x m. Input the numbers in first (n-1) x (m-1) cells. Find and place the sum of each row and each column in corresponding cells of last column and last row respectively. Finally, display the array elements along with the sum of rows and columns.
Sample Input
10 15 16 18 15 14 12 11 11 12 16 17 12 10 14 16 Sample Output
10 15 16 18 59 15 14 12 11 52 11 12 16 17 56 12 10 14 16 52 48 51 58 62 Write a program in Java to store the elements in two different double dimensional arrays (in matrix form) A and B each of order 4 x 4. Find the product of both the matrices and store the result in matrix C. Display the elements of matrix C.
Note:
Two matrixes can be multiplied only if the number of columns of the first matrix must be equal to the number of rows of the second matrix.Sample Input: Matrix A
3 2 1 2 6 4 5 0 7 -1 0 2 4 3 1 1 Sample Input: Matrix B
-2 -4 -1 0 3 6 -5 2 5 3 4 6 0 -2 2 5 Sample Output: Matrix C
5 -1 -5 20 25 15 -6 38 -17 -38 2 -8 6 3 -13 17 Write a Program in Java to fill a 2D array with the first 'mxn' prime numbers, where 'm' is the number of rows and 'n' is the number of columns.
For example:
If rows = 4 and columns = 5, then the result should be:2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 - Write a program to declare a square matrix A[ ][ ] of order N (N<20).
- Allow the user to input positive integers into this matrix. Perform the following tasks on the matrix.
- Output the original matrix.
- Find the SADDLE POINT for the matrix. A saddle point is an element of the matrix such that it is the minimum element for the row and the maximum element for the column to which it belongs. Saddle point for a given matrix is always unique. If the matrix has no saddle point, output the message "NO SADDLE POINT".
- If the matrix has a saddle point element then sort the elements of the left diagonal in ascending order using insertion sort technique. All other elements should remain unchanged.
Test your program for the following data and some random data:
Sample data:
Input:
n = 4Matrix A[ ][ ] 2 5 6 9 8 4 12 3 6 7 3 1 12 24 2 11 Output:
Matrix A[ ][ ] 2 5 6 9 8 4 12 3 6 7 3 1 12 24 2 11 No Saddle Point
Matrix after sorting the Principal diagonal:
2 5 6 9 8 3 12 3 6 7 4 1 12 24 2 11 Input:
n = 3Matrix A[ ][ ] 4 6 12 2 8 14 1 3 6 Output:
Matrix A[ ][ ] 4 6 12 2 8 14 1 3 6 Saddle Point = 4
Matrix after sorting the Principal diagonal:
4 6 12 2 6 14 1 3 8