Computer Science
Write a program in Java to enter natural numbers in a double dimensional array mxn (where m is the number of rows and n is the number of columns). Shift the elements of 4th column into the 1st column, the elements of 1st column into the 2nd column and so on. Display the new matrix.
11 | 16 | 7 | 4 |
8 | 10 | 9 | 18 |
9 | 8 | 12 | 15 |
14 | 15 | 13 | 6 |
Sample Input |
4 | 11 | 16 | 7 |
18 | 8 | 10 | 9 |
15 | 9 | 8 | 12 |
6 | 14 | 15 | 13 |
Sample Output |
Java
Java Arrays
17 Likes
Answer
import java.util.Scanner;
public class KboatSDAColShift
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number of rows (m): ");
int m = in.nextInt();
System.out.print("Enter number of columns (n): ");
int n = in.nextInt();
int arr[][] = new int[m][n];
int newArr[][] = new int[m][n];
System.out.println("Enter array elements");
for (int i = 0; i < m; i++) {
System.out.println("Enter Row "+ (i+1) + " :");
for (int j = 0; j < n; j++) {
arr[i][j] = in.nextInt();
}
}
System.out.println("Input Array:");
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
for (int j = 0; j < n; j++) {
int col = j + 1;
if (col == n) {
col = 0;
}
for (int i = 0; i < m; i++) {
newArr[i][col] = arr[i][j];
}
}
System.out.println("New Shifted Array:");
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
System.out.print(newArr[i][j] + "\t");
}
System.out.println();
}
}
}
Output
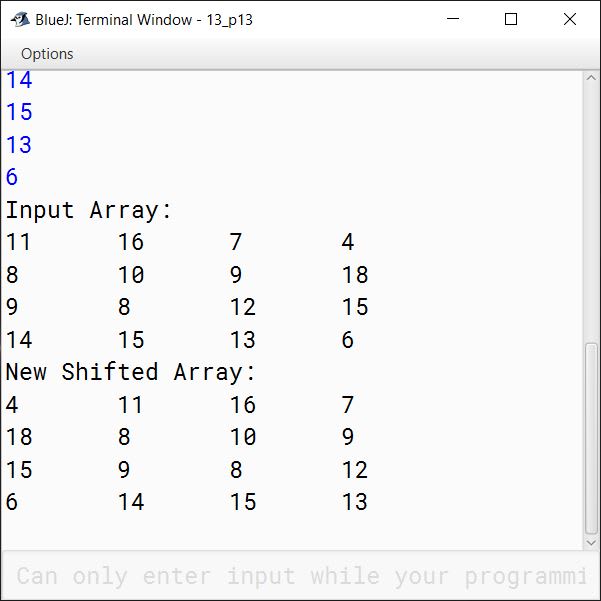
Answered By
4 Likes
Related Questions
A square matrix is the matrix in which number of rows equals the number of columns. Thus, a matrix of order n*n is called a Square Matrix.
Write a program in Java to create a double dimensional array of size nxn matrix form and fill the cells of matrix in a circular fashion (clock wise) with natural numbers from 1 to n2, taking n as an input. Input n should be an odd number and filling of the elements should start from the central cell.
For example, if n = 5, then n2 = 25, then the array is filled as:
21 22 23 24 25 20 7 8 9 10 19 6 1 2 11 18 5 4 3 12 17 16 15 14 13 Write a program in Java create a double dimensional array of size nxn matrix form and fill the numbers in a circular fashion (anticlock-wise) with natural numbers from 1 to n2, as illustrated below:
For example, if n = 5, then n2 = 25, then the array is filled as:
21 20 19 18 17 22 7 6 5 16 23 8 1 4 15 24 9 2 3 14 25 10 11 12 13 Write a program in Java to enter natural numbers in a double dimensional array m x n (where m is the number of rows and n is the number of columns). Display the new matrix in such a way that the new matrix is the mirror image of the original matrix.
8 15 9 18 9 10 7 6 10 8 11 13 12 16 17 19 Sample Input 18 9 15 8 6 7 10 9 13 11 8 10 19 17 16 12 Sample Output Write a Program in Java to fill a 2D array with the first 'mxn' prime numbers, where 'm' is the number of rows and 'n' is the number of columns.
For example:
If rows = 4 and columns = 5, then the result should be:2 3 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71