Computer Applications
Write a program to compute and display factorials of numbers between p and q where p > 0, q > 0, and p > q.
Java
Java Nested for Loops
2 Likes
Answer
import java.util.Scanner;
public class KboatFactRange
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter p: ");
int p = in.nextInt();
System.out.print("Enter q: ");
int q = in.nextInt();
if (p > q && p > 0 && q > 0) {
for (int i = q; i <= p; i++) {
long fact = 1;
for (int j = 1; j <= i; j++)
fact *= j;
System.out.println("Factorial of " + i +
" = " + fact);
}
}
else {
System.out.println("Invalid Input");
}
}
}
Output
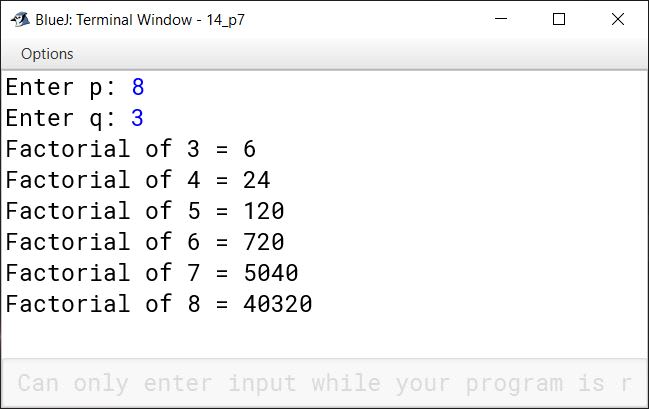
Answered By
2 Likes
Related Questions
What will be the value of sum after each of the following nested loops is executed?
int sum = 0; for (int i = 1; i <= 3; i++) for (int j = 1; j <= 3; j++) sum = sum + (i + j);
A happy number is a number which eventually reaches 1 when replaced by the sum of the square of each digit. For example, consider the number 320.
32 + 22 + 02 ⇒ 9 + 4 + 0 = 13
12 + 32 ⇒ 1 + 9 = 10
12 + 02 ⇒ 1 + 0 = 1Hence, 320 is a Happy Number.
Write a program in Java to enter a number and check if it is a Happy Number or not.
Write a program to generate a triangle or an inverted triangle till n terms based upon the user's choice.
Example 1:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 1
Enter the number of terms 5
Sample Output:
1
2 2
3 3 3
4 4 4 4
5 5 5 5 5Example 2:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 2
Enter the number of terms 6
Sample Output:
6 6 6 6 6 6
5 5 5 5 5
4 4 4 4
3 3 3
2 2
1Write a menu driven program that prompts the user to select one of the four triangle patterns (a, b, c, or d). The program then accepts number of rows and prints the selected pattern as shown below:
Enter the size : 8
(a)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(b)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(c)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(d)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #