Computer Applications
A happy number is a number which eventually reaches 1 when replaced by the sum of the square of each digit. For example, consider the number 320.
32 + 22 + 02 ⇒ 9 + 4 + 0 = 13
12 + 32 ⇒ 1 + 9 = 10
12 + 02 ⇒ 1 + 0 = 1
Hence, 320 is a Happy Number.
Write a program in Java to enter a number and check if it is a Happy Number or not.
Java
Java Iterative Stmts
48 Likes
Answer
import java.util.Scanner;
public class KboatHappyNumber
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter number to check: ");
long num = in.nextLong();
long sum = 0;
long n = num;
do {
sum = 0;
while (n != 0) {
int d = (int)(n % 10);
sum += d * d;
n /= 10;
}
n = sum;
} while (sum > 6);
if (sum == 1) {
System.out.println(num + " is a Happy Number");
}
else {
System.out.println(num + " is not a Happy Number");
}
}
}
Output
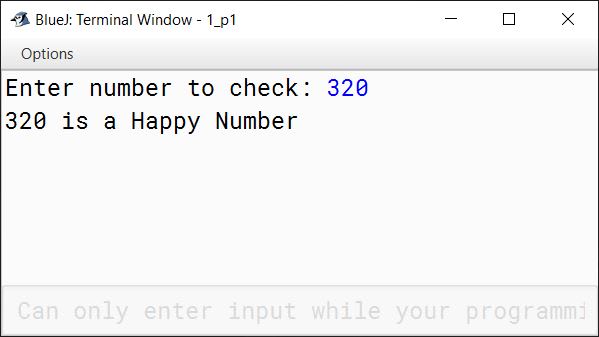
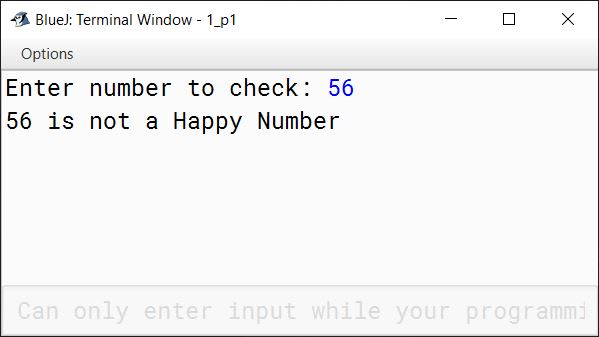
Answered By
16 Likes