Computer Applications
Write a menu driven program that prompts the user to select one of the four triangle patterns (a, b, c, or d). The program then accepts number of rows and prints the selected pattern as shown below:
Enter the size : 8
(a)
#
# #
# # #
# # # #
# # # # #
# # # # # #
# # # # # # #
# # # # # # # #
(b)
# # # # # # # #
# # # # # # #
# # # # # #
# # # # #
# # # #
# # #
# #
#
(c)
# # # # # # # #
# # # # # # #
# # # # # #
# # # # #
# # # #
# # #
# #
#
(d)
#
# #
# # #
# # # #
# # # # #
# # # # # #
# # # # # # #
# # # # # # # #
Java
Java Nested for Loops
3 Likes
Answer
import java.util.Scanner;
public class KboatTriangleChoice
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type a for Pattern a");
System.out.println("Type b for Pattern b");
System.out.println("Type c for Pattern c");
System.out.println("Type d for Pattern d");
System.out.print("Enter your choice: ");
char ch = in.next().charAt(0);
System.out.print("Enter the number of terms: ");
int n = in.nextInt();
switch (ch) {
case 'a':
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= i; j++)
System.out.print('#');
System.out.println();
}
break;
case 'b':
for (int i = n; i >= 1; i--) {
for (int j = 1; j <= i; j++)
System.out.print('#');
System.out.println();
}
break;
case 'c':
for (int i = n; i >= 1; i--) {
for (int j = i; j < n; j++)
System.out.print(" ");
for (int k = 0; k < i; k++)
System.out.print('#');
System.out.println();
}
break;
case 'd':
for (int i = n; i >= 1; i--) {
for (int j = 1; j < i; j++)
System.out.print(" ");
for (int k = i; k <= n; k++)
System.out.print('#');
System.out.println();
}
break;
default:
System.out.println("Incorrect choice");
break;
}
}
}
Output
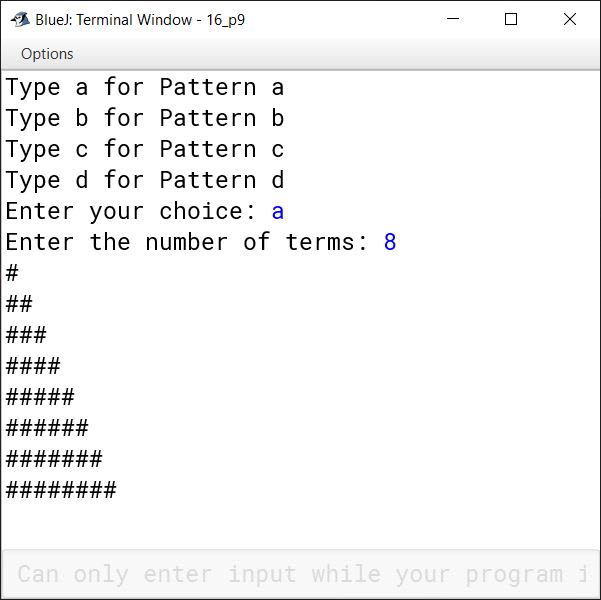
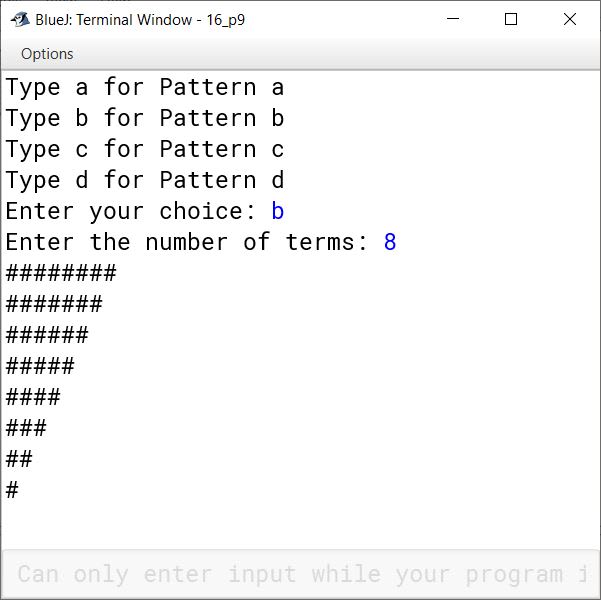
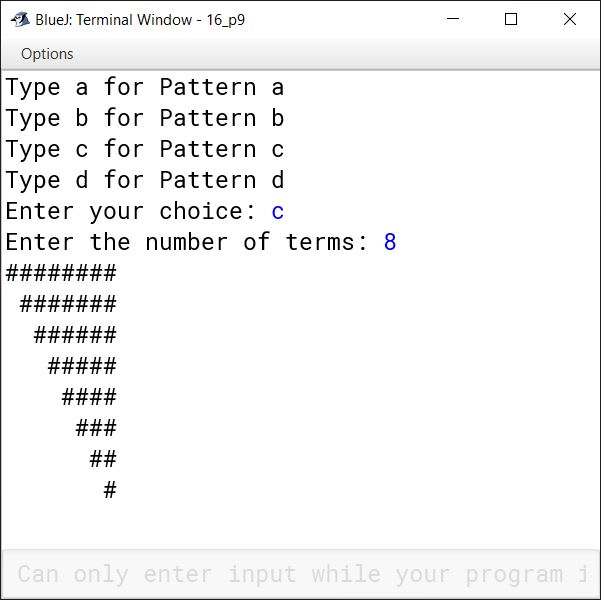
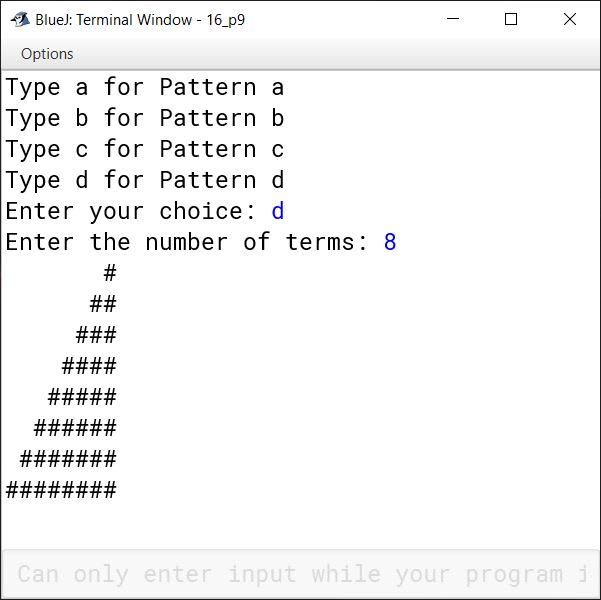
Answered By
3 Likes
Related Questions
Write a program to compute and display factorials of numbers between p and q where p > 0, q > 0, and p > q.
Write a program that computes sin x and cos x by using the following power series:
sin x = x - x3/3! + x5/5! - x7/7! + ……
cos x = 1 - x2/2! + x4/4! - x6/6! + ……
A happy number is a number which eventually reaches 1 when replaced by the sum of the square of each digit. For example, consider the number 320.
32 + 22 + 02 ⇒ 9 + 4 + 0 = 13
12 + 32 ⇒ 1 + 9 = 10
12 + 02 ⇒ 1 + 0 = 1Hence, 320 is a Happy Number.
Write a program in Java to enter a number and check if it is a Happy Number or not.
Write a menu driven program that prompts the user to select one of the four triangle patterns (a, b, c or d). The program then accepts number of rows and prints the selected pattern as shown below:
Enter the size: 8
(a)
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 1 2 3 4 5 6 7 1 2 3 4 5 6 7 8
(b)
1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 1 2 3 4 5 6 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1
(c)
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 1 2 3 4 5 6 7 1 2 3 4 5 6 7 8
(d)
1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 1 2 3 4 5 6 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1