Computer Applications
Write a program that computes sin x and cos x by using the following power series:
sin x = x - x3/3! + x5/5! - x7/7! + ……
cos x = 1 - x2/2! + x4/4! - x6/6! + ……
Java
Java Nested for Loops
6 Likes
Answer
import java.util.Scanner;
public class KboatSeries
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter x: ");
int x = in.nextInt();
double sinx = 0, cosx = 0;
int a = 1;
for (int i = 1; i <= 20; i += 2) {
long f = 1;
for (int j = 1; j <= i; j++)
f *= j;
double t = Math.pow(x, i) / f * a;
sinx += t;
a *= -1;
}
a = 1;
for (int i = 0; i <= 20; i += 2) {
long f = 1;
for (int j = 1; j <= i; j++)
f *= j;
double t = Math.pow(x, i) / f * a;
cosx += t;
a *= -1;
}
System.out.println("Sin x = " + sinx);
System.out.println("Cos x = " + cosx);
}
}
Output
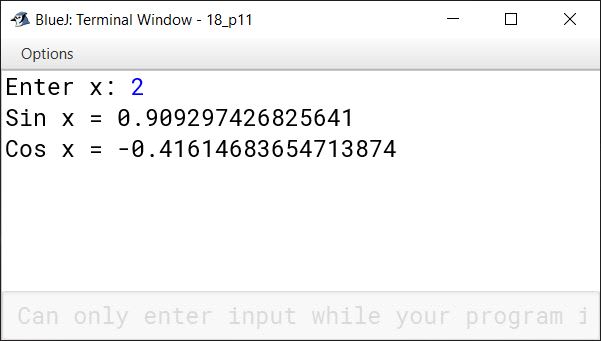
Answered By
2 Likes
Related Questions
Write a program to compute and display factorials of numbers between p and q where p > 0, q > 0, and p > q.
A happy number is a number which eventually reaches 1 when replaced by the sum of the square of each digit. For example, consider the number 320.
32 + 22 + 02 ⇒ 9 + 4 + 0 = 13
12 + 32 ⇒ 1 + 9 = 10
12 + 02 ⇒ 1 + 0 = 1Hence, 320 is a Happy Number.
Write a program in Java to enter a number and check if it is a Happy Number or not.
Write a menu driven program that prompts the user to select one of the four triangle patterns (a, b, c or d). The program then accepts number of rows and prints the selected pattern as shown below:
Enter the size: 8
(a)
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 1 2 3 4 5 6 7 1 2 3 4 5 6 7 8
(b)
1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 1 2 3 4 5 6 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1
(c)
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5 1 2 3 4 5 6 1 2 3 4 5 6 7 1 2 3 4 5 6 7 8
(d)
1 2 3 4 5 6 7 8 1 2 3 4 5 6 7 1 2 3 4 5 6 1 2 3 4 5 1 2 3 4 1 2 3 1 2 1
Write a menu driven program that prompts the user to select one of the four triangle patterns (a, b, c, or d). The program then accepts number of rows and prints the selected pattern as shown below:
Enter the size : 8
(a)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(b)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(c)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #
(d)
# # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # # #