Computer Applications
Write a program in Java to store 20 different names and telephone numbers of your friends in two different Single Dimensional Arrays (SDA). Now arrange all the names in alphabetical order and display all the names along with their respective telephone numbers using selection sort technique.
Java
Java String Handling
43 Likes
Answer
import java.util.Scanner;
public class KboatTelephoneBook
{
public static void main(String args[]) {
final int SIZE = 20;
Scanner in = new Scanner(System.in);
String names[] = new String[SIZE];
long telNos[] = new long[SIZE];
System.out.println("Enter " + SIZE + " names and telephone numbers");
for (int i = 0; i < SIZE; i++) {
System.out.print("Enter Name: ");
names[i] = in.nextLine();
System.out.print("Enter telephone number: ");
telNos[i] = in.nextLong();
in.nextLine();
}
//Selection Sort
for (int i = 0; i < SIZE - 1; i++) {
int min = i;
for (int j = i + 1; j < SIZE; j++) {
if (names[j].compareToIgnoreCase(names[min]) < 0) {
min = j;
}
}
String temp = names[min];
names[min] = names[i];
names[i] = temp;
long t = telNos[min];
telNos[min] = telNos[i];
telNos[i] = t;
}
System.out.println("Name\tTelephone Number");
for (int i = 0; i < SIZE; i++) {
System.out.println(names[i] + "\t" + telNos[i]);
}
}
}
Variable Description Table
Program Explanation
Output
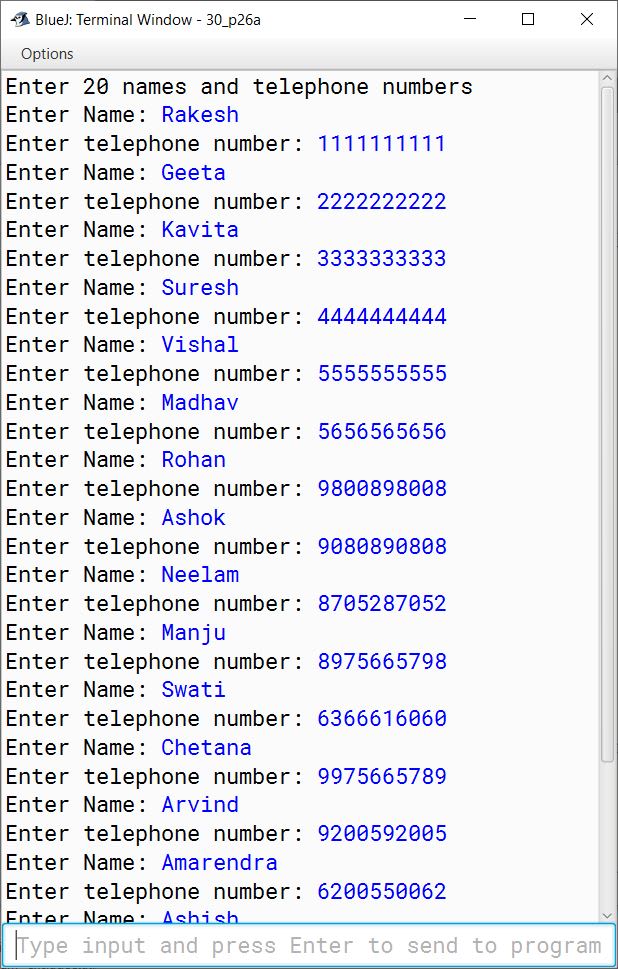
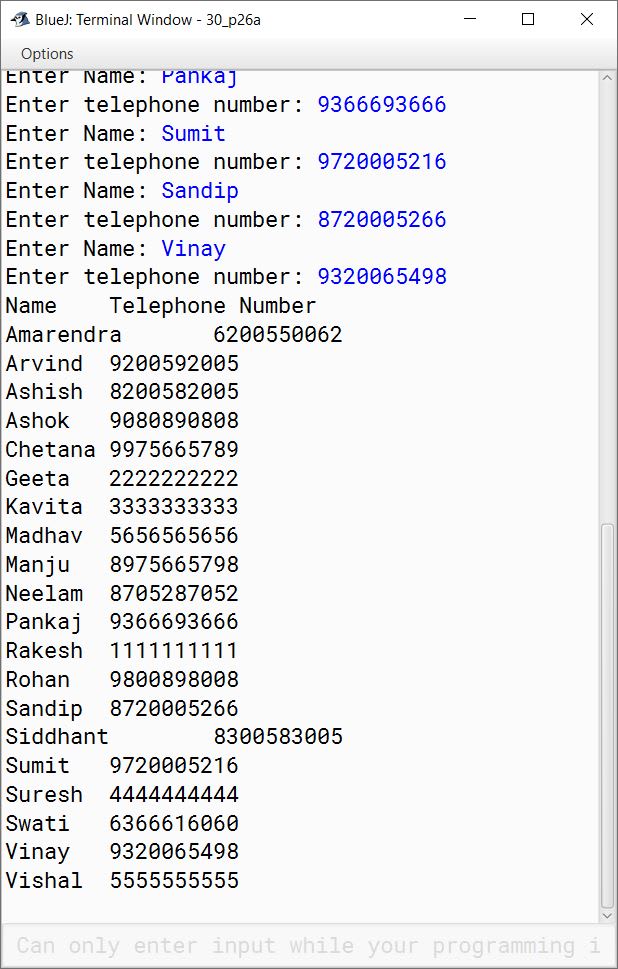
Answered By
11 Likes
Related Questions
Write a program in Java to store 10 different country names and their capitals in two different Single Dimensional Arrays (SDA). Display the country names (that starts with a vowel) along with their capitals in the given format.
Country Names Capital
xxxx xxxx
xxxx xxxxWrite a program to accept 10 names in a Single Dimensional Array (SDA). Display the names whose first letter matches with the letter entered by the user.
Sample Input:
Aman Shahi
Akash Gupta
Suman Mishra
and so on ……….Sample Output:
Enter the alphabet: A
Aman Shahi
Akash Gupta
…..
…..Write a program to input twenty names in an array. Arrange these names in ascending order of letters, using the bubble sort technique.
Sample Input:
Rohit, Devesh, Indrani, Shivangi, Himanshu, Rishi, Piyush, Deepak, Abhishek, Kunal, …..
Sample Output:
Abhishek, Deepak, Devesh, Himanshu, Indrani, Kunal, Piyush, Rishi, Rohit, Shivangi, …..Using the switch statement, write a menu driven program for the following:
(a) To print the Floyd’s triangle:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15(b) To display the following pattern:
I
I C
I C S
I C S EFor an incorrect option, an appropriate error message should be displayed.