Computer Applications
Write a program to accept 10 names in a Single Dimensional Array (SDA). Display the names whose first letter matches with the letter entered by the user.
Sample Input:
Aman Shahi
Akash Gupta
Suman Mishra
and so on ……….
Sample Output:
Enter the alphabet: A
Aman Shahi
Akash Gupta
…..
…..
Java
Java String Handling
48 Likes
Answer
import java.util.Scanner;
public class KboatSDANames
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
String names[] = new String[10];
System.out.println("Enter 10 names");
for (int i = 0; i < names.length; i++) {
names[i] = in.nextLine();
}
System.out.print("Enter a letter: ");
char ch = in.next().charAt(0);
ch = Character.toUpperCase(ch);
for (int i = 0; i < names.length; i++) {
if (Character.toUpperCase(names[i].charAt(0)) == ch) {
System.out.println(names[i]);
}
}
}
}
Variable Description Table
Program Explanation
Output
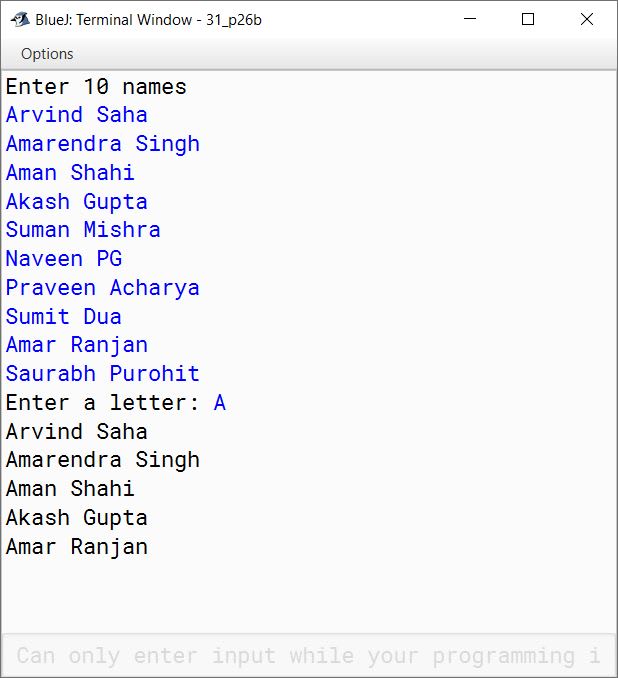
Answered By
16 Likes
Related Questions
Write a program in Java to store 10 different country names and their capitals in two different Single Dimensional Arrays (SDA). Display the country names (that starts with a vowel) along with their capitals in the given format.
Country Names Capital
xxxx xxxx
xxxx xxxxWrite a program in Java to store 20 different names and telephone numbers of your friends in two different Single Dimensional Arrays (SDA). Now arrange all the names in alphabetical order and display all the names along with their respective telephone numbers using selection sort technique.
Write a program in Java to input the names of 10 cities in a Single Dimensional Array. Display only those names which begin with a consonant but end with a vowel.
Sample Input: Kolkata, Delhi, Bengaluru, Jamshedpur, Bokaro, …….
Sample Output: Kolkata
Delhi
Bengaluru
Bokaro
….
….Write a program to input twenty names in an array. Arrange these names in ascending order of letters, using the bubble sort technique.
Sample Input:
Rohit, Devesh, Indrani, Shivangi, Himanshu, Rishi, Piyush, Deepak, Abhishek, Kunal, …..
Sample Output:
Abhishek, Deepak, Devesh, Himanshu, Indrani, Kunal, Piyush, Rishi, Rohit, Shivangi, …..