Computer Applications
Using the switch statement, write a menu driven program for the following:
(a) To print the Floyd’s triangle:
1
2 3
4 5 6
7 8 9 10
11 12 13 14 15
(b) To display the following pattern:
I
I C
I C S
I C S E
For an incorrect option, an appropriate error message should be displayed.
Java
Java String Handling
ICSE 2016
29 Likes
Answer
import java.util.Scanner;
public class KboatPattern
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.println("Type 1 for Floyd's triangle");
System.out.println("Type 2 for an ICSE pattern");
System.out.print("Enter your choice: ");
int ch = in.nextInt();
switch (ch) {
case 1:
int a = 1;
for (int i = 1; i <= 5; i++) {
for (int j = 1; j <= i; j++) {
System.out.print(a++ + "\t");
}
System.out.println();
}
break;
case 2:
String s = "ICSE";
for (int i = 0; i < s.length(); i++) {
for (int j = 0; j <= i; j++) {
System.out.print(s.charAt(j) + " ");
}
System.out.println();
}
break;
default:
System.out.println("Incorrect Choice");
}
}
}
Variable Description Table
Program Explanation
Output
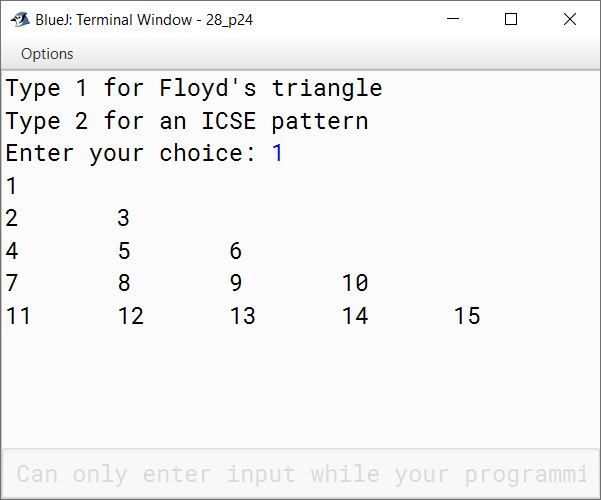
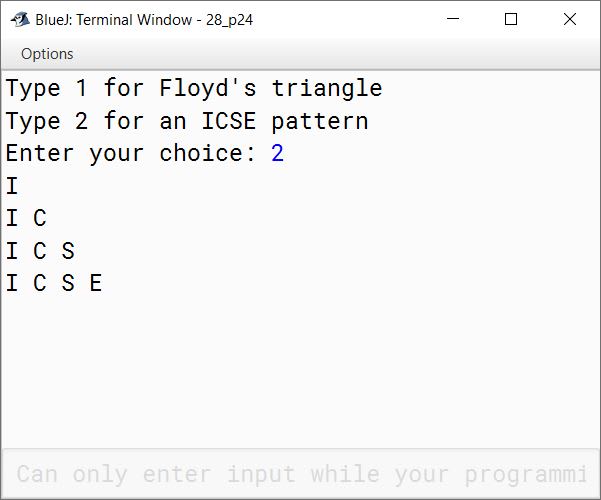
Answered By
9 Likes
Related Questions
Write a program to generate a triangle or an inverted triangle till n terms based upon the User’s choice of the triangle to be displayed.
Example 1:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 1
Enter the number of terms 5
Sample Output:* * * * * * * * * * * * * * *
Example 2:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 2
Enter the number of terms 5
Sample Output:A B C D E A B C D A B C A B A
Write a program to generate a triangle or an inverted triangle based upon User’s choice.
Example 1:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 1
Enter a word : BLUEJ
Sample Output:
B
L L
U U U
E E E E
J J J J JExample 2:
Input: Type 1 for a triangle and
Type 2 for an inverted triangle
Enter your choice 2
Enter a word : BLUEJ
Sample Output:
B L U E J
B L U E
B L U
B L
BWrite a program in Java to store 10 different country names and their capitals in two different Single Dimensional Arrays (SDA). Display the country names (that starts with a vowel) along with their capitals in the given format.
Country Names Capital
xxxx xxxx
xxxx xxxxWrite a program in Java to store 20 different names and telephone numbers of your friends in two different Single Dimensional Arrays (SDA). Now arrange all the names in alphabetical order and display all the names along with their respective telephone numbers using selection sort technique.