Computer Science
Write a Program in Java to input elements in a 2-D square matrix and check whether it is a Scalar Matrix or not.
Scalar Matrix: A scalar matrix is a diagonal matrix where the left diagonal elements are same.
The Matrix is:
5 0 0 0
0 5 0 0
0 0 5 0
0 0 0 5
The Matrix is Scalar
Java
Java Arrays
7 Likes
Answer
import java.util.Scanner;
public class KboatScalarMatrix
{
public static void main(String args[]) {
Scanner in = new Scanner(System.in);
System.out.print("Enter the size of the matrix: ");
int n = in.nextInt();
int arr[][] = new int[n][n];
System.out.println("Enter elements of the matrix: ");
for (int i = 0; i < n; i++) {
System.out.println("Enter Row "+ (i+1) + " :");
for (int j = 0; j < n; j++) {
arr[i][j] = in.nextInt();
}
}
System.out.println("The Matrix is:");
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
boolean isScalar = true;
int x = arr[0][0];
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if ((i == j && arr[i][j] != x)
|| ( i != j && arr[i][j] != 0)) {
isScalar = false;
break;
}
}
if (!isScalar) {
break;
}
}
if (isScalar) {
System.out.println("The Matrix is Scalar");
}
else {
System.out.println("The Matrix is not Scalar");
}
}
}
Output
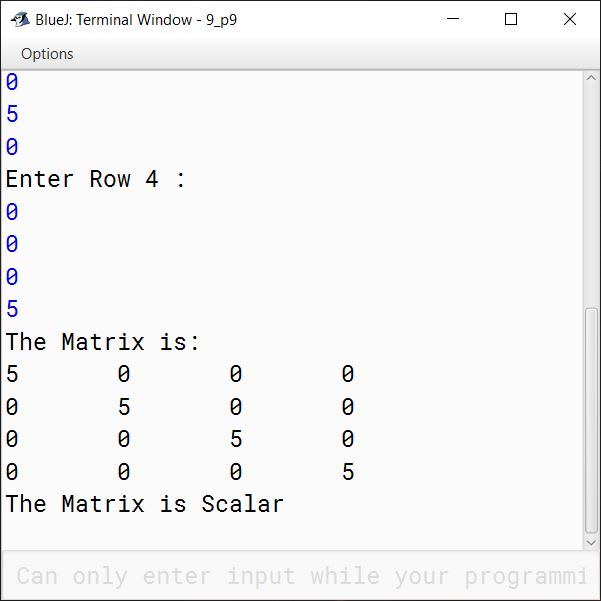
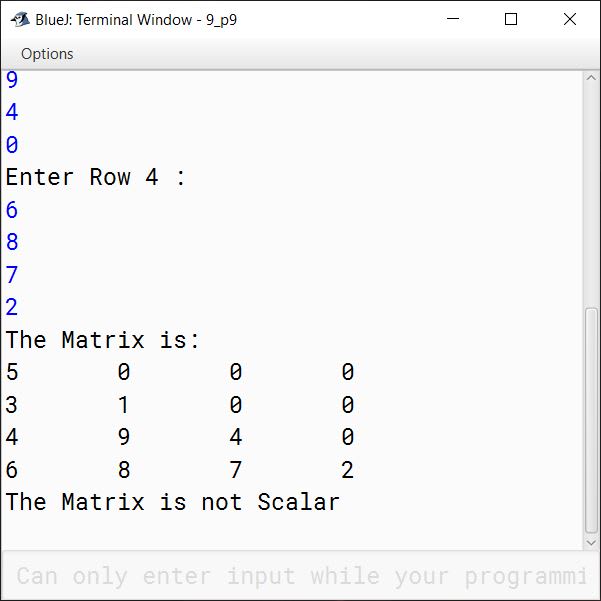
Answered By
1 Like
Related Questions
A square matrix is the matrix in which number of rows equals the number of columns. Thus, a matrix of order n*n is called a Square Matrix.
Write a program in Java to create a double dimensional array of size nxn matrix form and fill the numbers in a circular fashion (anticlock-wise) with natural numbers from 1 to n2, taking n as an input. The filling of the elements should start from outer to the central cell.
For example, if n=4, then n2=16, then the array is filled as:
1 ↓
↓ 12←
11←
102 ↓ ↓ 13 16 ↑ 9 ↑ 3 ↓ 14
→15 ↑
8 ↑ 4
→5
→6
→7 ↑
A square matrix is the matrix in which number of rows equals the number of columns. Thus, a matrix of order n*n is called a Square Matrix.
Write a program in Java to create a double dimensional array of size nxn matrix form and fill the cells of matrix in a circular fashion (clock wise) with natural numbers from 1 to n2, taking n as an input. Input n should be an odd number and filling of the elements should start from the central cell.
For example, if n = 5, then n2 = 25, then the array is filled as:
21 22 23 24 25 20 7 8 9 10 19 6 1 2 11 18 5 4 3 12 17 16 15 14 13 The result of a quiz competition is to be prepared as follows:
The quiz has five questions with four multiple choices (A, B, C, D), with each question carrying 1 mark for the correct answer. Design a program to accept the number of participants N such that N must be greater than 3 and less than 11. Create a double-dimensional array of size (Nx5) to store the answers of each participant row-wise. Calculate the marks for each participant by matching the correct answer stored in a single-dimensional array of size 5. Display the scores for each participant and also the participant(s) having the highest score.
Example: If the value of N = 4, then the array would be:
Q1 Q2 Q3 Q4 Q5 Participant 1 A B B C A Participant 2 D A D C B Participant 3 A A B A C Participant 4 D C C A B Key to the question: D C C B A Note: Array entries are line fed (i.e. one entry per line)
Test your program for the following data and some random data.
Example 1
INPUT:
N = 5
Participant 1 D A B C C
Participant 2 A A D C B
Participant 3 B A C D B
Participant 4 D A D C B
Participant 5 B C A D D
Key: B C D A AOUTPUT:
Scores:
Participant 1 = 0
Participant 2 = 1
Participant 3 = 1
Participant 4 = 1
Participant 5 = 2
Highest Score:
Participant 5Example 2
INPUT:
N = 4
Participant 1 A C C B D
Participant 2 B C A A C
Participant 3 B C B A A
Participant 4 C C D D B
Key: A C D B BOUTPUT:
Scores:
Participant 1 = 3
Participant 2 = 1
Participant 3 = 1
Participant 4 = 3
Highest Score:
Participant 1
Participant 4Example 3
INPUT:
N = 12OUTPUT:
INPUT SIZE OUT OF RANGE.Write a Program in Java to input elements in a 2D square matrix and check whether it is a Lower Triangular Matrix or not.
Lower Triangular Matrix: A Lower Triangular matrix is a square matrix in which all the entries above the main diagonal [] are zero. The entries below or on the main diagonal must be non zero values.
Enter the size of the matrix: 4
The Matrix is:
5 0 0 0
3 1 0 0
4 9 4 0
6 8 7 2The Matrix is Lower Triangular